Google Draco点云压缩 JS 实现
时间: 2023-10-10 19:04:41 浏览: 92
Google Draco点云压缩的JS实现需要使用Draco的JavaScript解码器,可以通过以下步骤实现:
1. 首先,从Draco的GitHub仓库下载JavaScript解码器,将解码器文件添加到你的项目中。
2. 加载点云数据,可以使用JavaScript的fetch API或XMLHttpRequest API来加载点云文件。
3. 创建Draco解码器对象,使用Draco解码器将加载的点云数据进行解码,生成一个Draco几何体对象。
4. 将Draco几何体对象转换为WebGL缓冲区对象,以便在WebGL中渲染。
下面是一个简单的示例代码:
```javascript
// 加载点云数据
fetch('point_cloud.drc')
.then(response => response.arrayBuffer())
.then(buffer => {
// 创建Draco解码器对象
const decoder = new DracoDecoderModule();
const decoderModule = decoder({});
const dracoDecoder = new decoderModule.Decoder();
// 解码点云数据
const dracoGeometry = new decoderModule.Mesh();
const bufferGeometry = new decoderModule.DecoderBuffer();
bufferGeometry.Init(new Int8Array(buffer), buffer.byteLength);
const geometryType = dracoDecoder.GetEncodedGeometryType(bufferGeometry);
if (geometryType == decoderModule.TRIANGULAR_MESH) {
dracoDecoder.DecodeBufferToMesh(bufferGeometry, dracoGeometry);
} else {
console.error('Unsupported geometry type');
}
decoderModule.destroy(bufferGeometry);
decoderModule.destroy(dracoDecoder);
// 将Draco几何体对象转换为WebGL缓冲区对象
const numPoints = dracoGeometry.num_points();
const numFaces = dracoGeometry.num_faces();
const indicesArray = new Uint32Array(numFaces * 3);
const positionsArray = new Float32Array(numPoints * 3);
const normalsArray = new Float32Array(numPoints * 3);
const indicesAttr = dracoGeometry.GetTrianglesUInt32Array();
const positionsAttr = dracoGeometry.GetAttributeByType(0, decoderModule.POSITION);
const normalsAttr = dracoGeometry.GetAttributeByType(0, decoderModule.NORMAL);
for (let i = 0; i < numFaces; i++) {
const index = i * 3;
indicesArray[index] = indicesAttr.GetValue(i * 3);
indicesArray[index + 1] = indicesAttr.GetValue(i * 3 + 1);
indicesArray[index + 2] = indicesAttr.GetValue(i * 3 + 2);
}
for (let i = 0; i < numPoints; i++) {
const index = i * 3;
const pos = positionsAttr.GetValue(i);
positionsArray[index] = pos[0];
positionsArray[index + 1] = pos[1];
positionsArray[index + 2] = pos[2];
const normal = normalsAttr.GetValue(i);
normalsArray[index] = normal[0];
normalsArray[index + 1] = normal[1];
normalsArray[index + 2] = normal[2];
}
decoderModule.destroy(dracoGeometry);
decoderModule.destroy(decoder);
// 在WebGL中渲染点云
// ...
});
```
在上面的代码中,我们使用fetch API加载点云数据,创建Draco解码器对象并解码点云数据,然后将Draco几何体对象转换为WebGL缓冲区对象,最后在WebGL中渲染点云。请注意,这只是一个简单的示例,实际应用中可能需要根据具体情况进行修改。
阅读全文
相关推荐
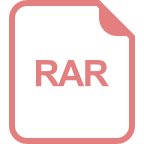
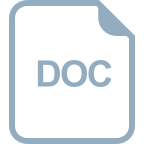
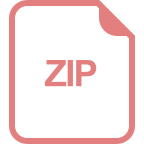
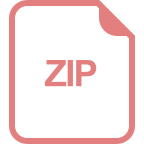
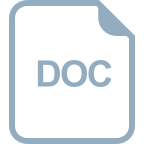
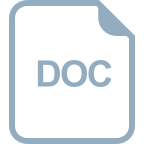
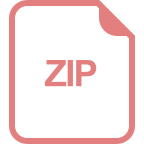
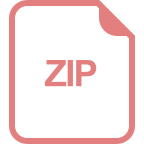
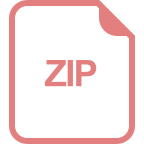
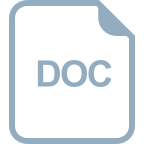
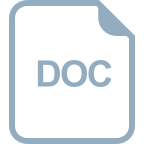

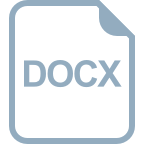
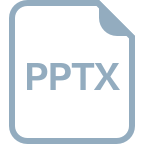
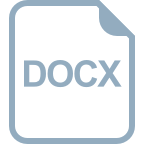