detectron2训练的模型怎么在windows环境下使用C++语言调用,并给出使用例子
时间: 2024-02-28 07:53:23 浏览: 73
在 Windows 环境下使用 C++ 调用 detectron2 训练的模型,需要先将模型转换成 ONNX 格式,然后使用 ONNX Runtime 进行推理。下面是一个简单的使用 detectron2 训练的 Mask R-CNN 模型进行推理的 C++ 代码例子。
首先,我们需要安装 ONNX Runtime。可以通过以下命令使用 pip 安装:
```
pip install onnxruntime
```
然后,我们需要将 detectron2 训练的 Mask R-CNN 模型转换成 ONNX 格式。可以使用以下代码:
```python
import torch
import onnx
from detectron2.modeling import build_model
from detectron2.checkpoint import DetectionCheckpointer
# 加载 detectron2 训练的模型
model = build_model(cfg) # cfg 为模型的配置文件
DetectionCheckpointer(model).load(path_to_model_weights) # path_to_model_weights 为模型权重文件路径
# 导出 ONNX 模型
dummy_input = torch.randn(batch_size, 3, img_height, img_width)
input_names = ["input"]
output_names = ["output"]
onnx.export(model, dummy_input, "model.onnx", input_names=input_names, output_names=output_names)
```
在导出 ONNX 模型时,需要指定输入和输出的名称。
然后,我们可以使用以下 C++ 代码进行推理:
```c++
#include <onnxruntime_cxx_api.h>
#include <opencv2/opencv.hpp>
int main() {
// 初始化 ONNX Runtime 环境
Ort::Env env(ORT_LOGGING_LEVEL_WARNING, "example");
// 加载 ONNX 模型
Ort::SessionOptions session_options;
Ort::Session session(env, "model.onnx", session_options);
// 获取输入和输出的名称
char* input_name = session.GetInputName(0, allocator);
char* output_name = session.GetOutputName(0, allocator);
// 加载输入数据
cv::Mat image = cv::imread("image.jpg");
cv::cvtColor(image, image, cv::COLOR_BGR2RGB);
cv::resize(image, image, cv::Size(img_width, img_height));
std::vector<float> input_data(image.data, image.data + image.total() * image.channels());
// 创建输入 tensor
Ort::MemoryInfo memory_info = Ort::MemoryInfo::CreateCpu(OrtDeviceAllocator, OrtMemTypeCPU);
std::vector<int64_t> input_shape = {batch_size, 3, img_height, img_width};
Ort::Value input_tensor = Ort::Value::CreateTensor<float>(memory_info, input_data.data(), input_data.size(), input_shape.data(), input_shape.size());
// 进行推理
std::vector<const char*> input_names = {input_name};
std::vector<const char*> output_names = {output_name};
std::vector<Ort::Value> input_tensors = {input_tensor};
std::vector<Ort::Value> output_tensors = session.Run(Ort::RunOptions{nullptr}, input_names.data(), input_tensors.data(), input_names.size(), output_names.data(), output_names.size());
// 获取输出 tensor
Ort::Value& output_tensor = output_tensors.front();
float* output_data = output_tensor.GetTensorMutableData<float>();
std::vector<int64_t> output_shape = output_tensor.GetTensorTypeAndShapeInfo().GetShape();
int num_classes = output_shape[1];
int output_height = output_shape[2];
int output_width = output_shape[3];
// 处理输出数据
cv::Mat output_image(output_height, output_width, CV_8UC3, cv::Scalar(0, 0, 0));
for (int y = 0; y < output_height; y++) {
for (int x = 0; x < output_width; x++) {
float max_score = output_data[0 * output_height * output_width + y * output_width + x];
int max_class = 0;
for (int c = 1; c < num_classes; c++) {
float score = output_data[c * output_height * output_width + y * output_width + x];
if (score > max_score) {
max_score = score;
max_class = c;
}
}
cv::Vec3b color = colors[max_class];
output_image.at<cv::Vec3b>(y, x) = color;
}
}
// 显示结果
cv::imshow("output", output_image);
cv::waitKey(0);
return 0;
}
```
在这个例子中,我们将输入图像调整为模型输入大小,并将其转换为 ONNX Runtime 中的输入 tensor。然后,我们调用 ONNX Runtime 的 Run 方法进行推理。最后,我们将输出 tensor 转换为图像,并显示结果。
需要注意的是,这个例子中使用了 OpenCV 库来加载和显示图像。如果你没有安装 OpenCV,可以使用其他库来代替。
阅读全文
相关推荐
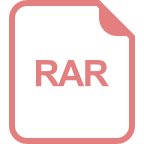
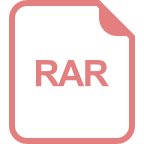
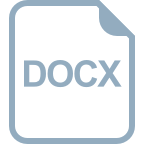
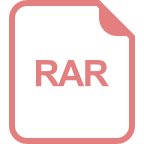
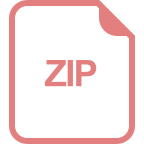
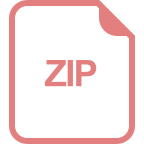
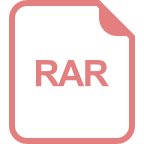
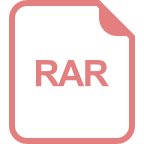
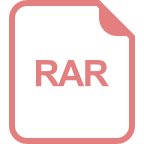
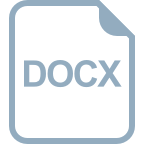
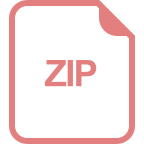
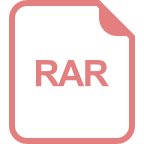
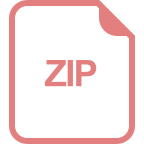
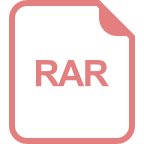
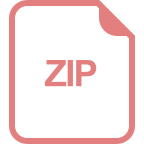
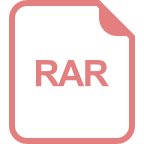