python遗传算法代码
时间: 2023-09-02 18:05:36 浏览: 84
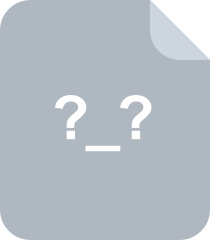
遗传算法python代码
以下是一个简单的 Python 遗传算法代码的示例:
```python
import random
# 遗传算法参数
POPULATION_SIZE = 100
GENERATIONS = 50
MUTATION_RATE = 0.1
# 问题参数
TARGET = 371
NUM_GENES = 6
GENE_VALUES = [i for i in range(10)]
def fitness(individual):
"""
计算个体的适应度
"""
return abs(sum(individual) - TARGET)
def generate_individual():
"""
生成随机个体
"""
return [random.choice(GENE_VALUES) for _ in range(NUM_GENES)]
def crossover(parent1, parent2):
"""
交叉操作
"""
point = random.randint(1, NUM_GENES-1)
return parent1[:point] + parent2[point:], parent2[:point] + parent1[point:]
def mutate(individual):
"""
变异操作
"""
for i in range(NUM_GENES):
if random.random() < MUTATION_RATE:
individual[i] = random.choice(GENE_VALUES)
return individual
# 初始化种群
population = [generate_individual() for _ in range(POPULATION_SIZE)]
# 开始迭代
for generation in range(GENERATIONS):
# 计算每个个体的适应度
fitness_scores = [fitness(individual) for individual in population]
# 找到适应度最好的个体
best_individual = population[fitness_scores.index(min(fitness_scores))]
print("Generation {}: Best individual = {}, Fitness = {}".format(generation, best_individual, fitness(best_individual)))
# 选择操作
parents = random.choices(population, weights=[1/fitness_score for fitness_score in fitness_scores], k=2)
# 交叉操作
child1, child2 = crossover(parents[0], parents[1])
# 变异操作
child1 = mutate(child1)
child2 = mutate(child2)
# 将子代加入种群
population += [child1, child2]
# 选择新的种群
population = sorted(population, key=lambda individual: fitness(individual))[:POPULATION_SIZE]
```
这段代码实现了一个简单的数字求和问题的遗传算法。在每个迭代中,我们计算每个个体的适应度,找到适应度最好的个体,然后进行选择、交叉和变异操作来生成新的子代。最后,我们将子代加入种群,并选择新的种群。在每个迭代结束时,我们打印出最佳个体的适应度和基因序列。
阅读全文
相关推荐
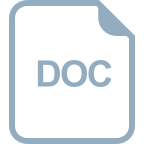

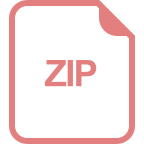
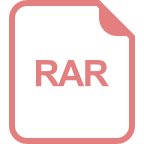
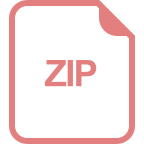
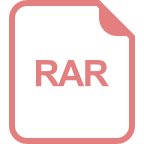
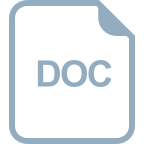
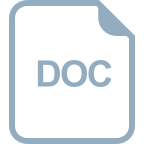
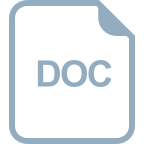




