用Java写一个生成一个学生对象Student对象有学号,年龄,身高。将学生对象存储到文件,然后从文件中读取数据重新生成Student对象。
时间: 2024-02-23 22:58:45 浏览: 20
好的,我可以为您提供一个大致的实现思路:
1. 定义一个名为`Student`的类,其中包含学号、年龄和身高三个属性,以及相应的 get/set 方法。
2. 使用 Java 的 IO 流将学生对象存储到文件。具体步骤如下:
- 创建`File`对象,指定文件路径和文件名。
- 创建`FileOutputStream`对象,将`File`对象作为参数传入。
- 创建`ObjectOutputStream`对象,将`FileOutputStream`对象作为参数传入。
- 使用`writeObject()`方法将学生对象写入输出流,并使用`flush()`方法刷新输出流。
- 关闭输出流。
3. 从文件中读取数据重新生成`Student`对象。具体步骤如下:
- 创建`File`对象,指定文件路径和文件名。
- 创建`FileInputStream`对象,将`File`对象作为参数传入。
- 创建`ObjectInputStream`对象,将`FileInputStream`对象作为参数传入。
- 使用`readObject()`方法从输入流中读取学生对象,并强制转换为`Student`类型。
- 关闭输入流。
下面是一个大致的代码实现:
```java
import java.io.*;
public class Student implements Serializable {
private int id;
private int age;
private double height;
public Student(int id, int age, double height) {
this.id = id;
this.age = age;
this.height = height;
}
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
public double getHeight() {
return height;
}
public void setHeight(double height) {
this.height = height;
}
public static void main(String[] args) {
Student student = new Student(1001, 18, 1.75);
// 写入文件
try {
FileOutputStream fos = new FileOutputStream("student.dat");
ObjectOutputStream oos = new ObjectOutputStream(fos);
oos.writeObject(student);
oos.flush();
oos.close();
fos.close();
} catch (IOException e) {
e.printStackTrace();
}
// 读取文件
try {
FileInputStream fis = new FileInputStream("student.dat");
ObjectInputStream ois = new ObjectInputStream(fis);
Student s = (Student) ois.readObject();
System.out.println(s.getId() + ", " + s.getAge() + ", " + s.getHeight());
ois.close();
fis.close();
} catch (IOException | ClassNotFoundException e) {
e.printStackTrace();
}
}
}
```
当然,这只是一个简单的示例,实际应用中可能需要考虑更多的异常处理以及文件路径的动态获取等问题。
相关推荐
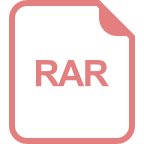
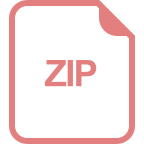
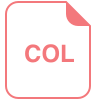
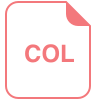
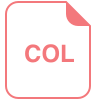
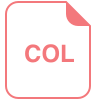
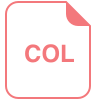









