俄罗斯方块Python代码
时间: 2023-09-05 20:05:14 浏览: 164
### 回答1:
俄罗斯方块是一款经典的游戏,它可以用多种编程语言来实现。如果你是问关于用Python实现俄罗斯方块的代码,以下是一个简单的实现方式:
```
import turtle
# 定义砖块大小和颜色
block_size = 20
colors = ['red', 'yellow', 'blue', 'green', 'purple', 'orange']
# 初始化窗口
turtle.setup(width=600, height=600)
turtle.screensize(600, 600)
turtle.speed(0)
# 循环生成砖块
for color in colors:
turtle.color(color)
for i in range(4):
turtle.forward(block_size)
turtle.right(90)
turtle.penup()
turtle.forward(2 * block_size)
turtle.pendown()
# 等待窗口关闭
turtle.done()
```
这仅仅是一个简单的实现,实际上俄罗斯方块游戏有很多复杂的部分,包括方块的移动、旋转、掉落等,这些部分需要更多的代码来实现。
### 回答2:
俄罗斯方块是一款经典的益智游戏,Python可以通过GUI编程库来实现这个游戏。
首先,需要导入必要的库,如tkinter用于创建游戏界面和事件处理等。然后,创建一个窗口对象,并设置窗口大小和标题。
接着,需要定义一些常量,如方块的大小、窗口的大小、方块的形状等等。可以将这些常量存储在一个字典中,以便于后续使用。
然后,需要定义一些函数来处理游戏逻辑,如生成方块、移动方块、旋转方块、消除方块等等。在生成方块的函数中,可以使用随机数来决定生成的方块的形状和颜色。
接下来,需要定义一个主循环来处理用户的输入和更新游戏界面。在主循环中,可以通过事件处理来监听用户的按键事件,并调用相应的函数来移动、旋转或下落方块。同时,可以在每次更新游戏界面时检测方块是否触底或与其他方块发生碰撞,如果发生碰撞,则将该方块固定在游戏界面上,并生成下一个方块。
最后,可以在主循环外创建一个线程用于定时更新游戏界面,以模拟方块的下落。可以使用time.sleep()函数来控制定时器的速度。
以上是一个简单的俄罗斯方块Python代码的基本框架。具体的代码细节可以根据个人的需求和想法进行编写和调整,如添加游戏得分和关卡、优化界面显示等等。希望以上回答对您有所帮助。
### 回答3:
俄罗斯方块是一款经典的益智游戏,玩家需要操作下落的四方块,使其在游戏区域内拼出完整的一行或多行,被消除后将获得得分。以下是一个简单的实现俄罗斯方块的Python代码:
```python
import pygame
import random
# 游戏区域大小
screen_width = 800
screen_height = 600
# 方块大小和颜色
block_size = 30
colors = [(0, 0, 0), (255, 0, 0), (0, 255, 0), (0, 0, 255), (255, 255, 0), (255, 0, 255), (0, 255, 255), (128, 128, 128)]
# 方块形状
tetrominoes = [
[[1, 1, 1, 1]],
[[1, 1], [1, 1]],
[[1, 1, 1], [0, 1, 0]],
[[1, 1, 1], [1, 0, 0]],
[[1, 1, 1], [0, 0, 1]],
[[1, 1, 0], [0, 1, 1]],
[[0, 1, 1], [1, 1, 0]]
]
def draw_block(x, y, color):
pygame.draw.rect(screen, color, (x, y, block_size, block_size))
def draw_board(board):
for y in range(len(board)):
for x in range(len(board[y])):
draw_block(x * block_size, y * block_size, colors[board[y][x]])
def check_collision(board, tetromino, x, y):
for row in range(len(tetromino)):
for col in range(len(tetromino[row])):
if tetromino[row][col] and (y + row >= len(board) or x + col < 0 or x + col >= len(board[y])) or board[y + row][x + col]:
return True
return False
def rotate_tetromino(tetromino):
return list(zip(*tetromino[::-1]))
def clear_lines(board):
lines_cleared = 0
y = len(board) - 1
while y >= 0:
if all(board[y]):
del board[y]
lines_cleared += 1
else:
y -= 1
for _ in range(lines_cleared):
board.insert(0, [0] * len(board[0]))
return lines_cleared
def main():
pygame.init()
screen = pygame.display.set_mode((screen_width, screen_height))
clock = pygame.time.Clock()
board = [[0] * (screen_width // block_size) for _ in range(screen_height // block_size)]
current_tetromino = random.choice(tetrominoes)
x, y = screen_width // (2 * block_size) - len(current_tetromino[0]) // 2, 0
score = 0
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
return
keys = pygame.key.get_pressed()
if keys[pygame.K_LEFT] and not check_collision(board, current_tetromino, x - 1, y):
x -= 1
if keys[pygame.K_RIGHT] and not check_collision(board, current_tetromino, x + 1, y):
x += 1
if keys[pygame.K_DOWN] and not check_collision(board, current_tetromino, x, y + 1):
y += 1
if keys[pygame.K_UP]:
rotated = rotate_tetromino(current_tetromino)
if not check_collision(board, rotated, x, y):
current_tetromino = rotated
if not check_collision(board, current_tetromino, x, y + 1):
y += 1
else:
for row in range(len(current_tetromino)):
for col in range(len(current_tetromino[row])):
if current_tetromino[row][col]:
board[y + row][x + col] = 1
score += clear_lines(board)
current_tetromino = random.choice(tetrominoes)
x, y = screen_width // (2 * block_size) - len(current_tetromino[0]) // 2, 0
screen.fill((255, 255, 255))
draw_board(board)
for row in range(len(current_tetromino)):
for col in range(len(current_tetromino[row])):
if current_tetromino[row][col]:
draw_block((x + col) * block_size, (y + row) * block_size, colors[1])
pygame.display.update()
clock.tick(10)
if __name__ == '__main__':
main()
```
这个代码使用了`pygame`库来创建游戏窗口和处理用户输入,基本思路是不断地根据用户输入以及当前方块的状态更新游戏区域,利用二维列表来表示方块的状态以及游戏区域的状态,通过检测碰撞和消除完整的一行来实现游戏规则。
阅读全文
相关推荐
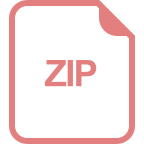
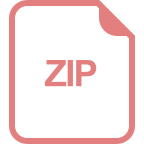
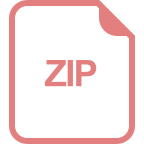
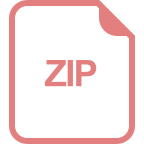
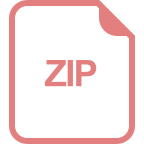
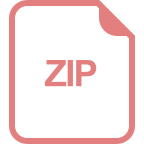
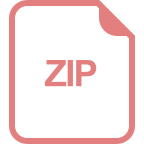
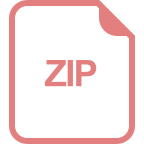
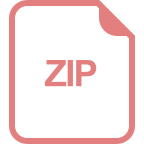
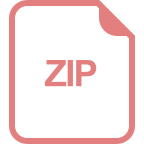
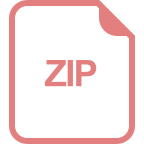