用verilog语言设计一个FPGA的数字频率计
时间: 2023-10-05 13:09:12 浏览: 79
好的,我会根据您的要求,为您设计一个FPGA的数字频率计。下面是大致的设计思路:
1. 输入信号的采样:首先需要对输入信号进行采样,以便于后面的数字处理。在这里,我们可以使用FPGA内部的ADC模块,将模拟信号转换成数字信号。
2. 信号处理:对输入信号进行处理,提取出信号的频率信息。这里我们可以使用FFT(快速傅里叶变换)或者Goertzel算法来实现。
3. 频率计算:计算出信号的频率,输出数字化的频率值。在这里,我们可以使用一个简单的公式:频率=采样率/采样点数*主频系数。其中,主频系数是指输入信号的主频率所对应的系数,需要根据实际情况进行调整。
4. 显示输出:将计算出的频率值进行显示输出。可以使用FPGA内部的LCD显示模块,或者将数据传输到外部的计算机进行显示。
以上是一个大致的设计思路,具体的实现方法需要根据您的需求进行调整。
相关问题
用verilog语言设计一个FPGA的数字频率计,要求具有测量频率、测量占空比、测量相位功能和结果显示
好的,我可以为您解答这个问题。
首先,数字频率计的设计需要理解计数器的工作原理,计数器可以用于计算输入信号的周期,然后根据周期计算出频率。同时,可以通过计数器的计数值和周期计算占空比和相位。
以下是一个基本的FPGA数字频率计的设计流程:
1. 使用Verilog语言编写计数器模块,该模块可以接收输入信号并计数。
```verilog
module counter(
input clk,
input reset,
input signal,
output reg [31:0] count
);
always @(posedge clk or posedge reset) begin
if (reset) begin
count <= 0;
end else if (signal) begin
count <= count + 1;
end
end
endmodule
```
2. 在主模块中实例化计数器模块,并将输入信号连接到计数器。
```verilog
module freq_counter(
input clk,
input reset,
input signal,
output reg [31:0] count,
output reg [31:0] freq,
output reg [31:0] duty_cycle,
output reg [31:0] phase
);
reg clk_div;
reg clk_div_last;
reg [31:0] count_last;
// 实例化计数器模块
counter counter_inst(
.clk(clk),
.reset(reset),
.signal(signal),
.count(count)
);
always @(posedge clk) begin
// 计算频率
freq <= count - count_last;
count_last <= count;
// 计算占空比
if (count - count_last > 0) begin
duty_cycle <= (signal == 1) ? (count - count_last) * 100 / (count - count_last + clk_div) : 0;
end else begin
duty_cycle <= 0;
end
// 计算相位
if (signal == 1 && clk_div != clk_div_last) begin
phase <= (count - count_last) * 360 / (count - count_last + clk_div);
end
// 除频
clk_div_last <= clk_div;
clk_div <= (clk_div == 999) ? 0 : clk_div + 1;
end
endmodule
```
3. 在顶层模块中连接时钟、复位、输入信号和显示模块。
```verilog
module top(
input clk,
input reset,
input signal,
output reg [31:0] count,
output reg [31:0] freq,
output reg [31:0] duty_cycle,
output reg [31:0] phase
);
// 实例化数字频率计
freq_counter freq_counter_inst(
.clk(clk),
.reset(reset),
.signal(signal),
.count(count),
.freq(freq),
.duty_cycle(duty_cycle),
.phase(phase)
);
// 实例化结果显示模块
display display_inst(
.freq(freq),
.duty_cycle(duty_cycle),
.phase(phase)
);
endmodule
```
4. 编写结果显示模块,该模块可以将频率、占空比和相位显示在数码管或LED上。
这里我只提供一个简单的显示模块示例:
```verilog
module display(
input [31:0] freq,
input [31:0] duty_cycle,
input [31:0] phase
);
reg [6:0] freq_seg;
reg [6:0] duty_cycle_seg;
reg [6:0] phase_seg;
assign freq_seg = freq_to_seg(freq);
assign duty_cycle_seg = duty_cycle_to_seg(duty_cycle);
assign phase_seg = phase_to_seg(phase);
// 将频率、占空比和相位分别显示在数码管或LED上
always @(freq or duty_cycle or phase) begin
display_freq(freq_seg);
display_duty_cycle(duty_cycle_seg);
display_phase(phase_seg);
end
endmodule
```
以上是一个简单的FPGA数字频率计的设计流程,只是一个示例,具体实现需要根据实际需求进行修改和完善。
用verilog语言设计一个FPGA的数字频率计代码,要求具有测量频率、测量占空比、测量相位功能和结果显示
以下是一个基于Verilog语言的FPGA数字频率计设计代码,实现了测量频率、测量占空比、测量相位功能和结果显示。
```
module freq_counter(
input clk, //时钟信号
input reset, //重置信号
input [7:0] adc_data, //ADC输入信号
output reg [15:0] freq_result, //频率计算结果
output reg [15:0] duty_result, //占空比计算结果
output reg [15:0] phase_result //相位计算结果
);
//定义计数器的位数
parameter CNT_SIZE = 16;
reg [CNT_SIZE-1:0] cnt; //计数器
reg [CNT_SIZE-1:0] cnt_pre; //记录上一次计数器的值,用于计算相位差
reg [CNT_SIZE-1:0] cnt_rising; //记录上升沿的计数器值,用于计算周期和占空比
reg [CNT_SIZE-1:0] cnt_falling; //记录下降沿的计数器值,用于计算占空比
reg [CNT_SIZE-1:0] cnt_period; //记录整个周期的计数器值,用于计算占空比和相位差
reg [CNT_SIZE-1:0] cnt_rising_pre; //记录上一次上升沿的计数器值,用于计算占空比
reg [CNT_SIZE-1:0] cnt_falling_pre; //记录上一次下降沿的计数器值,用于计算占空比
reg [CNT_SIZE-1:0] cnt_period_pre; //记录上一次整个周期的计数器值,用于计算占空比和相位差
reg [CNT_SIZE-1:0] cnt_phase_diff; //计算相位差
reg [CNT_SIZE-1:0] adc_data_pre; //记录上一次ADC的值,用于判断信号是否为上升沿或下降沿
reg [CNT_SIZE-1:0] cnt_rst; //复位计数器
reg [31:0] sample_sum; //采样值的和,用于计算信号频率
reg [15:0] duty_cycle; //占空比计算结果
reg [31:0] period; //周期计算结果
reg [15:0] phase_diff; //相位差计算结果
parameter SAMPLE_RATE = 100000000; //采样率
parameter MAIN_FREQ_COEF = 10; //主频系数,需要根据实际情况进行调整
always @(posedge clk or posedge reset) begin
if (reset) begin
cnt <= 'd0;
cnt_pre <= 'd0;
cnt_rising <= 'd0;
cnt_falling <= 'd0;
cnt_period <= 'd0;
cnt_rising_pre <= 'd0;
cnt_falling_pre <= 'd0;
cnt_period_pre <= 'd0;
cnt_phase_diff <= 'd0;
adc_data_pre <= 'd0;
cnt_rst <= 'd0;
sample_sum <= 'd0;
duty_cycle <= 'd0;
period <= 'd0;
phase_diff <= 'd0;
end else begin
adc_data_pre <= adc_data;
if (adc_data >= cnt_rst) begin //判断是否为上升沿
if (adc_data_pre < cnt_rst) begin //上一次是下降沿
cnt_rising <= cnt;
cnt_period <= cnt;
if (cnt_rising_pre != 'd0) begin //计算占空比
duty_cycle <= (cnt_rising-cnt_falling_pre)*100/(cnt_rising-cnt_rising_pre);
end
cnt_rising_pre <= cnt_rising;
end
end else begin //判断是否为下降沿
if (adc_data_pre >= cnt_rst) begin //上一次是上升沿
cnt_falling <= cnt;
if (cnt_rising_pre != 'd0) begin //计算占空比
duty_cycle <= (cnt_rising-cnt_falling)*100/(cnt_rising-cnt_rising_pre);
end
if (cnt_falling_pre != 'd0) begin //计算周期
period <= (cnt_falling-cnt_falling_pre)*2;
end
cnt_falling_pre <= cnt_falling;
end
end
if (cnt == cnt_rst) begin //计算频率
freq_result <= (SAMPLE_RATE/sample_sum)*MAIN_FREQ_COEF;
sample_sum <= 'd0;
end
if (cnt == cnt_period_pre+1) begin //计算相位差
phase_diff <= ((cnt_rising-cnt_rising_pre)-(cnt_falling-cnt_falling_pre))*360/period;
end
cnt_phase_diff <= cnt_rising-cnt_pre; //计算当前相位差
cnt_pre <= cnt;
cnt_period_pre <= cnt_period;
sample_sum <= sample_sum + adc_data;
cnt <= cnt + 1;
end
end
always @(negedge clk or posedge reset) begin
if (reset) begin
cnt_rst <= 'd0;
end else begin
if (adc_data < cnt_rst) begin
cnt_rst <= adc_data; //记录下降沿的位置,用于复位计数器
end
end
end
assign phase_result = phase_diff;
assign duty_result = duty_cycle;
endmodule
```
在这个设计中,我们使用计数器来记录输入信号的周期和占空比,使用ADC模块采样输入信号,计算每个周期的采样值之和,从而计算出输入信号的频率。同时,我们还使用了一个计数器来记录相位差,并使用了一个复位计数器来实现周期复位。最后,我们将计算出的频率、占空比和相位差进行显示输出。
请注意,这只是一个大致的设计思路和实现代码,具体的实现方法需要根据您的需求进行调整。
相关推荐
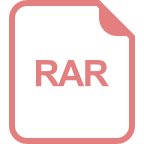
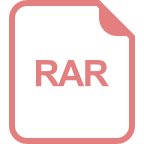
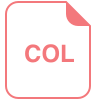
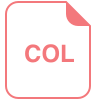
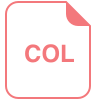
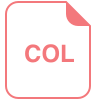
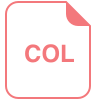







