package com.jy.common.netty.strategy.channelstrategy; import com.jy.common.netty.protobuf.Message; import com.jy.common.netty.service.ClientService; import com.jy.common.netty.strategy.ChannelReadStrategy; import com.jy.common.utils.spring.SpringUtils; import io.netty.channel.ChannelHandlerContext; import lombok.extern.slf4j.Slf4j; import org.springframework.stereotype.Component; /** * 储存MAC地址 */ @Component @Slf4j public class NormalStrategy implements ChannelReadStrategy { private Message.CommandType commandType = Message.CommandType.MAC_ADDRESS; private ClientService clientService; public ClientService getClientService() { if (clientService == null) { clientService = SpringUtils.getBean(ClientService.class); } return clientService; } @Override public void channelRead(ChannelHandlerContext ctx, Object t) { Message message= (Message) t; getClientService().add(message.getMac(),ctx); log.info("mac地址保存成功!"); } @Override public Message.CommandType getCommandType() { return commandType; } } 优化这段代码
时间: 2024-02-28 21:53:20 浏览: 142
1. 将 getClientService() 方法中的 if (clientService == null) {} 判断移动到成员变量声明处,并使用 @Autowired 注解进行依赖注入。
2. 在注入 ClientService 时,使用构造函数注入方式,避免在 getClientService() 中每次都从 Spring 容器中获取 Bean。
3. 将 @Component 注解移动到类名上方,方便阅读。
4. 使用 @Override 注解来标注重写的方法。
5. 将成员变量 commandType 的类型改为 final,避免在运行时被修改。
具体代码如下:
```java
package com.jy.common.netty.strategy.channelstrategy;
import com.jy.common.netty.protobuf.Message;
import com.jy.common.netty.service.ClientService;
import com.jy.common.netty.strategy.ChannelReadStrategy;
import lombok.extern.slf4j.Slf4j;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Component;
@Component
@Slf4j
public class NormalStrategy implements ChannelReadStrategy {
private final Message.CommandType commandType = Message.CommandType.MAC_ADDRESS;
private final ClientService clientService;
@Autowired
public NormalStrategy(ClientService clientService) {
this.clientService = clientService;
}
@Override
public void channelRead(ChannelHandlerContext ctx, Object t) {
Message message = (Message) t;
clientService.add(message.getMac(), ctx);
log.info("mac地址保存成功!");
}
@Override
public Message.CommandType getCommandType() {
return commandType;
}
}
```
阅读全文
相关推荐
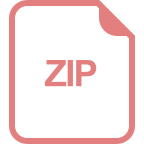
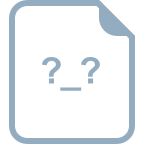

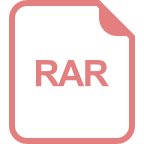
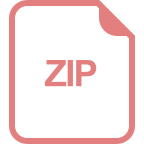
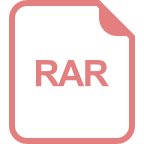
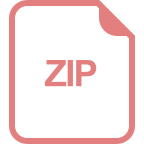
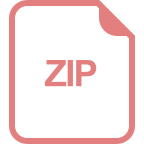
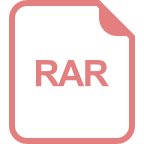
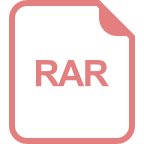
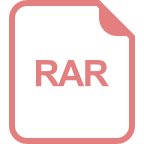
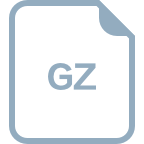
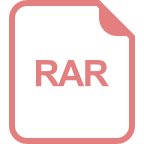
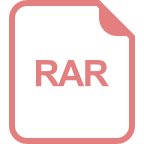
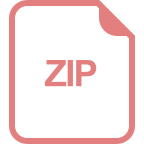
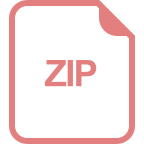
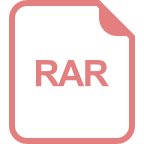