c语言实现DES加密算法代码
时间: 2024-11-19 17:15:46 浏览: 43
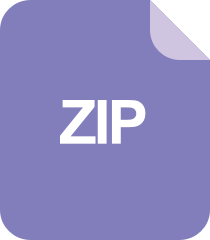
DES加密算法(c语言实现)
在C语言中,实现DES(Data Encryption Standard)加密算法通常涉及到使用OpenSSL库,因为DES是一种复杂的密码学算法,直接编写可能会非常复杂。以下是使用OpenSSL库的一个简化的示例,展示了如何对数据进行DES加密:
```c
#include <stdio.h>
#include <openssl/des.h>
// 数据块大小
#define DES_BLOCK_SIZE 8
void des_encrypt(char *plaintext, char *ciphertext) {
DES_KEY_schedule key;
unsigned char plaintext_pad[DES_BLOCK_SIZE], ciphertext_pad[DES_BLOCK_SIZE];
DES_cblock des_key;
// 预处理输入数据(填充PKCS5)
memset(plaintext_pad, 0, DES_BLOCK_SIZE);
plaintext_pad[DES_BLOCK_SIZE - 1] = 1; // 添加奇偶校验位
des_set_key(&key, (unsigned char*) "your_secret_key", DES_KEY_LENGTH); // 替换为实际密钥
DES_ecb_encrypt((unsigned char*) plaintext, ciphertext_pad, &key, DES_ENCRYPT);
// 结果需要再次预处理,移除填充和奇偶校验
for(int i = 0; i < DES_BLOCK_SIZE; i++) {
ciphertext[i] = ciphertext_pad[i] ^ plaintext_pad[i];
}
}
int main() {
char plaintext[] = "Hello, DES!";
char ciphertext[DES_BLOCK_SIZE * 2]; // 加密后的长度可能比明文长
des_encrypt(plaintext, ciphertext);
printf("Encrypted data: %s\n", ciphertext);
return 0;
}
```
注意,这只是一个基本的示例,实际应用中你需要处理错误、内存管理以及安全的关键存储等问题。此外,现代通信中DES已不再是最安全的选择,通常会使用更强大的算法如AES。
阅读全文
相关推荐
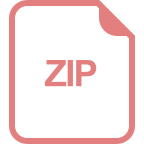
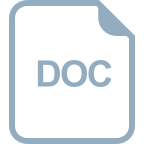
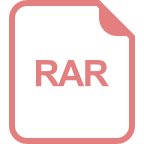
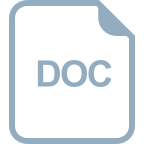
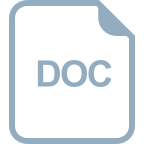
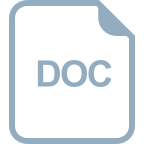
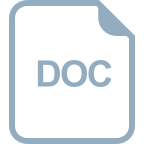




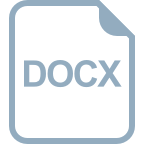
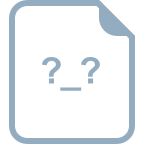