使用vue2加elementui使用无忧2.0+饿了蒙特组件库封装类似百度搜索栏的组件,要求包含防抖节流功能及数据实时展示
时间: 2024-01-21 19:19:17 浏览: 71
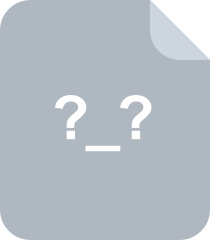
前端实用vue+elementui(前后端分离)
首先,需要安装 ElementUI 和无忧2.0组件库:
```
npm install element-ui
npm install @mengt/element-ui
```
然后,创建一个 SearchBox.vue 组件:
```vue
<template>
<el-input :value="keyword" @input="handleInput" placeholder="请输入关键字"></el-input>
</template>
<script>
import { debounce } from 'lodash'
export default {
name: 'SearchBox',
props: {
delay: {
type: Number,
default: 300
}
},
data () {
return {
keyword: ''
}
},
methods: {
handleInput: debounce(function (value) {
this.keyword = value
this.$emit('input', value)
}, this.delay)
}
}
</script>
```
这里使用了 lodash 库中的 debounce 函数来实现防抖功能。在用户输入时,会触发 handleInput 方法,该方法使用 debounce 函数对输入进行防抖处理,并将最终的关键字通过 $emit 发送给父组件。
接着,在父组件中使用 SearchBox 组件,并实时展示数据:
```vue
<template>
<div>
<SearchBox v-model="keyword" @input="search" />
<ul>
<li v-for="item in filteredData">{{ item }}</li>
</ul>
</div>
</template>
<script>
import { throttle } from 'lodash'
export default {
name: 'Demo',
data () {
return {
keyword: '',
data: ['apple', 'banana', 'orange', 'pear', 'grape']
}
},
computed: {
filteredData () {
return this.data.filter(item => item.includes(this.keyword))
}
},
methods: {
search: throttle(function (value) {
console.log('search', value)
}, 1000)
}
}
</script>
```
在父组件中,使用 computed 属性对 data 进行筛选,只展示包含关键字的数据。同时,使用 throttle 函数来实现节流功能,减少搜索请求的次数。
最后,将父组件渲染到页面中:
```vue
<template>
<div id="app">
<Demo />
</div>
</template>
<script>
import Demo from '@/components/Demo.vue'
export default {
name: 'App',
components: {
Demo
}
}
</script>
```
阅读全文
相关推荐
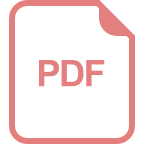
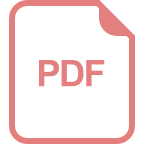
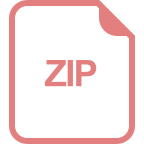
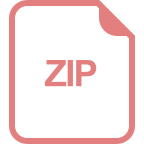
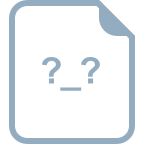
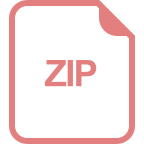
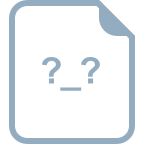
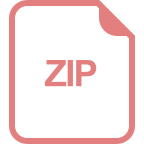
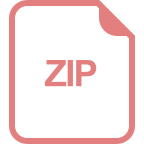
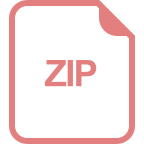
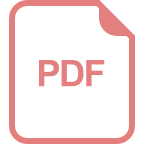
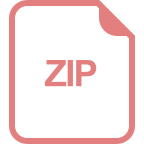
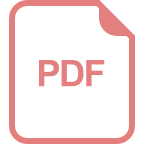
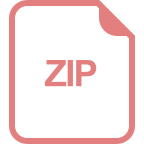
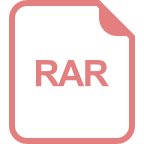
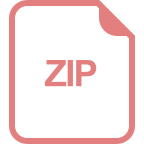
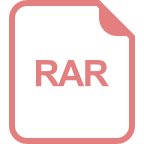