使用c sharp 向http server发送json文件的代码
时间: 2024-05-08 19:16:02 浏览: 11
以下是使用C#向HTTP服务器发送JSON文件的示例代码:
```csharp
using System;
using System.Net;
using System.IO;
using Newtonsoft.Json;
namespace HttpJsonTest
{
class Program
{
static void Main(string[] args)
{
// 创建HTTP请求
HttpWebRequest request = (HttpWebRequest)WebRequest.Create("https://yourdomain.com/api/data");
request.Method = "POST";
request.ContentType = "application/json";
// 准备要发送的数据
MyData data = new MyData()
{
Name = "John",
Age = 30,
Email = "john@example.com"
};
string json = JsonConvert.SerializeObject(data);
// 把数据写入请求体
using (StreamWriter writer = new StreamWriter(request.GetRequestStream()))
{
writer.Write(json);
writer.Flush();
}
// 发送请求并接收响应
HttpWebResponse response = (HttpWebResponse)request.GetResponse();
using (StreamReader reader = new StreamReader(response.GetResponseStream()))
{
string result = reader.ReadToEnd();
Console.WriteLine(result);
}
}
}
// 自定义数据类型
class MyData
{
public string Name { get; set; }
public int Age { get; set; }
public string Email { get; set; }
}
}
```
上面的代码中,我们使用`HttpWebRequest`类创建了一个HTTP请求,并设置了请求的方法为POST,内容类型为JSON。然后,我们准备了要发送的数据,并把它写入请求体中。最后,我们发送请求并接收响应,从响应体中读取了返回的数据。在这个例子中,我们使用了`Newtonsoft.Json`库来序列化数据为JSON格式。
相关推荐
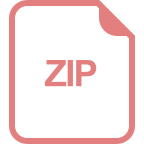
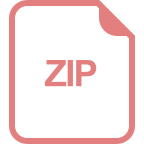
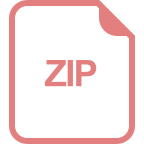














