编写jdbc,包含编写servlet类,service层,dao层,用statement语句实现查询,详细方法
时间: 2024-02-03 21:02:13 浏览: 30
好的,以下是一个详细的方法,用 Statement 语句实现查询,并包含了 DAO 层、Service 层和 Servlet 类。
1. 创建数据库连接
使用 JDBC API 创建一个数据库连接。你需要提供数据库的 URL、用户名和密码。
示例代码:
```
String url = "jdbc:mysql://localhost:3306/test";
String user = "root";
String password = "root";
Connection conn = DriverManager.getConnection(url, user, password);
```
2. 编写 DAO 层
在 DAO 层中,你需要实现对数据库的操作。在本例中,我们使用 Statement 对象执行查询语句,然后使用 ResultSet 对象处理查询结果。
示例代码:
```
public class UserDao {
private Connection conn;
public UserDao() {
// 在构造函数中获取数据库连接
String url = "jdbc:mysql://localhost:3306/test";
String user = "root";
String password = "root";
try {
conn = DriverManager.getConnection(url, user, password);
} catch (SQLException e) {
e.printStackTrace();
}
}
public List<User> getUsers() throws SQLException {
List<User> users = new ArrayList<>();
Statement stmt = null;
ResultSet rs = null;
try {
stmt = conn.createStatement();
rs = stmt.executeQuery("SELECT * FROM users");
while (rs.next()) {
User user = new User();
user.setId(rs.getInt("id"));
user.setName(rs.getString("name"));
user.setAge(rs.getInt("age"));
users.add(user);
}
} finally {
if (rs != null) {
rs.close();
}
if (stmt != null) {
stmt.close();
}
}
return users;
}
public void close() throws SQLException {
if (conn != null) {
conn.close();
}
}
}
```
3. 编写 Service 层
在 Service 层中,你需要调用 DAO 层的方法,并处理数据。在本例中,我们将查询结果转换为 JSON 格式,以便在 Servlet 中进行输出。
示例代码:
```
public class UserService {
private UserDao userDao;
public UserService() {
userDao = new UserDao();
}
public String getUsersJson() throws SQLException {
List<User> users = userDao.getUsers();
ObjectMapper objectMapper = new ObjectMapper();
String json = objectMapper.writeValueAsString(users);
return json;
}
public void close() throws SQLException {
userDao.close();
}
}
```
4. 编写 Servlet 类
在 Servlet 类中,你需要处理请求,并调用 Service 层的方法。在本例中,我们将查询结果输出为 JSON 格式。
示例代码:
```
public class UserServlet extends HttpServlet {
private UserService userService;
public UserServlet() {
userService = new UserService();
}
@Override
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
String json;
try {
json = userService.getUsersJson();
response.setContentType("application/json");
response.setCharacterEncoding("UTF-8");
response.getWriter().write(json);
} catch (SQLException e) {
e.printStackTrace();
}
}
@Override
public void destroy() {
// 在销毁方法中关闭数据库连接
try {
userService.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
}
```
在本例中,我们使用了 Servlet 3.0 的注解方式,不需要在 web.xml 中配置 Servlet。如果你使用的是 Servlet 2.5 或更早的版本,需要在 web.xml 中配置 Servlet。
以上是一个基本的 Java Web 使用 Statement 语句实现查询的方法,希望对你有帮助。请注意,以上代码仅供参考,具体实现可能需要根据你的具体情况进行修改。
相关推荐
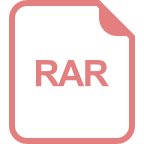
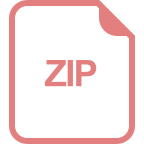
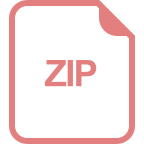
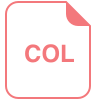
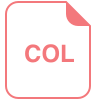
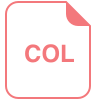
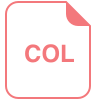
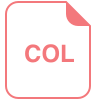









