vue-resource get 传递表单
时间: 2023-09-05 11:05:14 浏览: 63
### 回答1:
在 Vue.js 中使用 vue-resource 来进行 GET 请求并传递表单数据,可以使用 $http.get() 方法,并在请求的参数中传入表单数据。
示例:
```
this.$http.get('api/form', {
params: {
formData: this.form
}
}).then(response => {
// 处理响应
}, response => {
// 处理错误
});
```
其中,'api/form' 是请求的地址,this.form 是表单数据。
注意: 如果后端服务器不支持 GET 请求带参数,可以尝试使用 POST 或其他请求方式。
### 回答2:
在Vue.js中,可以通过`vue-resource`库来发送GET请求并传递表单数据。首先,您需要安装`vue-resource`库。可以通过以下命令来安装:
```
npm install vue-resource
```
安装完成后,在您的Vue.js项目中引入`vue-resource`:
```javascript
import Vue from 'vue'
import VueResource from 'vue-resource'
Vue.use(VueResource)
```
接下来,您可以在Vue组件中使用`this.$http.get`方法来发送GET请求。为了传递表单数据,您需要将表单数据作为参数传递给该方法。示例如下:
```javascript
this.$http.get('/api/data', { params: this.formData })
.then(response => {
// 处理响应
})
.catch(error => {
// 处理错误
})
```
上述示例中,`this.formData`是一个包含表单数据的对象。在GET请求中,`params`选项用于传递参数。`this.$http.get`方法返回一个Promise对象,您可以通过`.then`方法处理成功响应,通过`.catch`方法处理错误。
当GET请求发送时,`vue-resource`会将表单数据编码为URL参数并附在请求URL中。例如,如果`this.formData`为`{ name: 'John', age: 25 }`,那么请求URL将变为`/api/data?name=John&age=25`。
通过上述步骤,您可以使用`vue-resource`库进行GET请求并传递表单数据。
### 回答3:
Vue-resource 是一个基于 Vue.js 的网络请求库,它可以通过 GET 方法来传递表单数据。
使用 Vue-resource 的 GET 方法,可以通过设置请求的 URL 参数,来传递表单数据。首先,需要在 Vue 组件中引入 Vue-resource:
```javascript
import Vue from 'vue'
import VueResource from 'vue-resource'
Vue.use(VueResource)
```
然后,可以通过在组件的 methods 中定义一个发送 GET 请求的方法,来传递表单数据。例如:
```javascript
methods: {
sendData() {
// 接收表单数据
const formData = {
name: this.name,
age: this.age,
email: this.email
}
// 发送 GET 请求
this.$http.get('http://example.com/api/formData', formData).then(response => {
// 处理请求成功的响应
console.log(response.body)
}, error => {
// 处理请求失败的响应
console.error(error)
})
}
}
```
在上述代码中,sendData() 方法接收表单数据,然后通过 this.$http.get() 方法发送 GET 请求,同时将表单数据作为第二个参数传递进去。之后,根据请求的结果,可以在 then() 方法和 catch() 方法中处理成功或失败的响应。
需要注意的是,在发送 GET 请求时,表单数据是通过 URL 参数的方式进行传递的,所以需要根据实际情况在后端进行处理。如果需要传递较大的表单数据,建议使用 POST 方法,并将表单数据放在请求的 body 中进行传递。
相关推荐
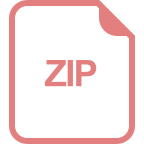
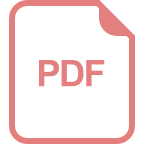
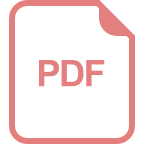














