为什么SpringCloud gateway 统一响应结果,response Body 为空时拦截不到
时间: 2023-03-25 15:02:00 浏览: 278
SpringCloud gateway 统一响应结果时,如果 response Body 为空,可能是因为响应头中的 Content-Type 与实际返回的数据类型不匹配,或者是后端服务返回的数据本身就为空。可以检查一下响应头和后端服务的返回数据,看看是否有问题。
相关问题
Spring Cloud Gateway 截获请求的body
Spring Cloud Gateway 是一个基于 Spring Framework 的轻量级、高性能的 API 网关,它允许开发者路由、过滤、增强和拦截网络流量,包括 HTTP 请求的 body 部分。
在 Spring Cloud Gateway 中,你可以通过 `WebFlux` 的响应拦截器机制来截获并处理请求的 body。具体步骤如下:
1. **创建拦截器**:首先,你需要自定义一个 `GlobalFilter` 或 `ExchangeFilterFunction` 类,这是 Gateway 提供的用于添加全局过滤功能的接口。
```java
import org.springframework.cloud.gateway.filter.GatewayFilter;
import org.springframework.cloud.gateway.filter.GatewayFilterFunction;
import org.springframework.stereotype.Component;
@Component
public class BodyLoggingFilter implements GatewayFilterFunction {
@Override
public GatewayFilter apply(String id) {
return new LoggingBodyGatewayFilter(id);
}
}
```
这里 `LoggingBodyGatewayFilter` 是一个具体的拦截器实现,它会记录请求的 body 内容。
2. **配置启用**:在 Gateway 的配置类(如 `application.yml` 或 `application.properties`)中注册这个过滤器,并指定需要应用的路径前缀。
```yaml
spring:
cloud:
gateway:
filters:
- Predicates:
path=/api/**
filter-factory: com.example.BodyLoggingFilter
```
这表示对所有路径以 `/api` 开头的请求都会应用你的拦截器。
3. **拦截逻辑**:在 `LoggingBodyGatewayFilter` 中,你可以获取到 `ServerWebExchange` 对象,从中读取请求体的内容,然后进行处理、日志记录等操作。
```java
@Component
public class LoggingBodyGatewayFilter extends ServerWebExchangeConverter {
private final String id;
public LoggingBodyGatewayFilter(String id) {
this.id = id;
}
@Override
protected Mono<Void> doFilter(ServerWebExchange exchange, WebFilterChain chain) {
return chain.filter(exchange).flatMap(response -> {
// 这里可以访问 request body
Object requestBody = exchange.getAttributes().get(BodyExtractor.REQUEST_BODY_ATTRIBUTE);
log.info("Received request with ID {} and body: {}", id, requestBody);
return response;
});
}
}
```
gateway3.1.1修改请求body内容
在Spring Cloud Gateway 3.1.1版本中,如果你想修改请求体的内容,你可以通过自定义`WebFilter`或者使用Spring MVC的拦截器来实现。以下是两种常见的方法:
1. **自定义WebFilter**:
- 首先,创建一个实现了`GlobalFilter`接口的类,并覆盖`filter`方法,这里你可以使用`ServerWebExchange`对象获取到`HttpHeaders`和`RequestBody`,对内容进行处理后再发送出去。
```java
@Bean
public GlobalFilter customBodyFilter() {
return (exchange, chain) -> {
ServerHttpRequest request = exchange.getRequest();
HttpHeaders headers = request.getHeaders();
// 修改或替换请求体内容
Mono<ServerHttpResponse> responseMono = exchange.filter(chain)
.flatMap(response -> {
if (headers.getContentType().toString().startsWith(MediaType.APPLICATION_JSON)) {
String requestBody = ... // 根据需要修改后的请求体
byte[] bytes = requestBody.getBytes(StandardCharsets.UTF_8);
HttpHeaders newHeaders = headers.set("Content-Length", String.valueOf(bytes.length));
return response.header(newHeaders).body(BodyInserters.fromBytes(bytes));
} else {
return response;
}
});
return responseMono;
};
}
```
2. **Spring MVC 拦截器**:
如果你的应用使用了Spring MVC,可以利用AOP(面向切面编程)技术,比如`HandlerInterceptor`来拦截并处理请求。在`preHandle()`方法中操作`HttpServletRequest`对象的`request.getParameterMap()`或`request.getInputStream()`。
在实际操作时,请确保你的安全策略、日志记录以及错误处理都已经考虑到位。
阅读全文
相关推荐
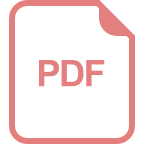
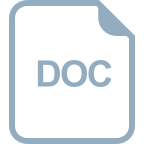
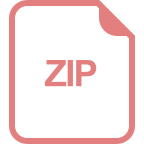
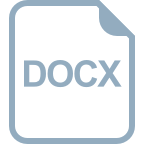
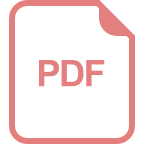
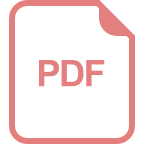
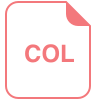
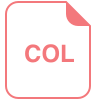
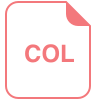
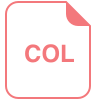
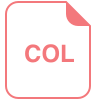
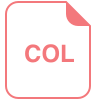
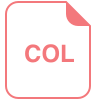
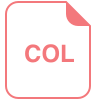
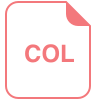
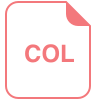
