C++代码编写:1.编写一个Date类,有checkMonth和checkDay函数以检查月份和日期的合法性,有判断是否为闰年的函数,设计定义set类函数,用以设定年,月份和日期,设计定义多个get函数用以获取年、月和日。 2. 创建一个抽象类employee类,该类中有姓名(sting 类型),ID(sting 类型)和出生日期(Date类型)。定义构造函数初始化每个成员;定义changeName()函数用来修改姓名,定义Display()函数用来显示每个员工的基本信息。纯虚函数为发放工资函数。 3.创建由Employee类派生出的其它四个员工类Salaried Employee,Commission Employee,Hourly Employee,以及由Commission Employee派生出的BasePlus Commission Employee。编写他们的新增成员、构造函数等,他们都有一个计算工资的函数。
时间: 2024-03-26 12:39:53 浏览: 24
1. Date类的代码如下:
```C++
class Date {
private:
int year;
int month;
int day;
bool isLeapYear() {
return (year % 4 == 0 && year % 100 != 0) || year % 400 == 0;
}
public:
Date(int y = 0, int m = 0, int d = 0) {
year = y;
month = m;
day = d;
}
bool checkMonth() {
return month >= 1 && month <= 12;
}
bool checkDay() {
if (month == 2) {
if (isLeapYear()) {
return day >= 1 && day <= 29;
} else {
return day >= 1 && day <= 28;
}
} else if (month == 4 || month == 6 || month == 9 || month == 11) {
return day >= 1 && day <= 30;
} else {
return day >= 1 && day <= 31;
}
}
void setYear(int y) {
year = y;
}
void setMonth(int m) {
month = m;
}
void setDay(int d) {
day = d;
}
int getYear() {
return year;
}
int getMonth() {
return month;
}
int getDay() {
return day;
}
};
```
2. Employee类的代码如下:
```C++
class Employee {
private:
string name;
string id;
Date birthDate;
public:
Employee(string n, string i, Date bd) {
name = n;
id = i;
birthDate = bd;
}
void changeName(string n) {
name = n;
}
void Display() {
cout << "Name: " << name << endl;
cout << "ID: " << id << endl;
cout << "Birth Date: " << birthDate.getYear() << "-" << birthDate.getMonth() << "-" << birthDate.getDay() << endl;
}
virtual double pay() = 0;
};
```
注意到这里声明了一个纯虚函数pay(),表示需要在派生出的具体类中实现。
3. SalariedEmployee类、CommissionEmployee类、HourlyEmployee类和BasePlusCommissionEmployee类的代码如下:
```C++
class SalariedEmployee : public Employee {
private:
double salary;
public:
SalariedEmployee(string n, string i, Date bd, double s) : Employee(n, i, bd) {
salary = s;
}
double pay() {
return salary;
}
};
class CommissionEmployee : public Employee {
private:
double sales;
double rate;
public:
CommissionEmployee(string n, string i, Date bd, double s, double r) : Employee(n, i, bd) {
sales = s;
rate = r;
}
double pay() {
return sales * rate;
}
};
class HourlyEmployee : public Employee {
private:
double wage;
double hours;
public:
HourlyEmployee(string n, string i, Date bd, double w, double h) : Employee(n, i, bd) {
wage = w;
hours = h;
}
double pay() {
if (hours <= 40) {
return wage * hours;
} else {
return wage * 40 + wage * 1.5 * (hours - 40);
}
}
};
class BasePlusCommissionEmployee : public CommissionEmployee {
private:
double baseSalary;
public:
BasePlusCommissionEmployee(string n, string i, Date bd, double s, double r, double b) : CommissionEmployee(n, i, bd, s, r) {
baseSalary = b;
}
double pay() {
return CommissionEmployee::pay() + baseSalary;
}
};
```
这里可以看到,派生类的构造函数中都直接调用了基类的构造函数,使用了冒号“:”语法。同时,派生类中的pay()函数都实现了基类中声明的纯虚函数。
相关推荐
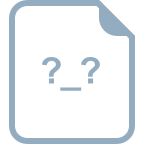
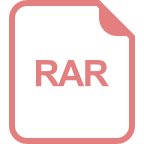














