用python编写程序统计1行字符串中: 不同字符的个数和每种字符出现的次数。输出按升序排列出
时间: 2023-06-25 16:02:11 浏览: 94
可以使用 Python 中的字典(dict)来进行统计。
代码如下:
```python
str = input("请输入字符串:")
# 定义一个空的字典,用于存储每种字符出现的次数
char_dict = {}
# 遍历字符串,统计每种字符出现的次数
for char in str:
if char in char_dict:
char_dict[char] += 1
else:
char_dict[char] = 1
# 将字典按照键(即字符)进行升序排序
sorted_dict = sorted(char_dict.items(), key=lambda item:item[0])
# 输出不同字符的个数和每种字符出现的次数
print("不同字符的个数为:", len(sorted_dict))
print("每种字符出现的次数为:")
for item in sorted_dict:
print(item[0], ":", item[1])
```
运行程序后,输入一个字符串,即可得到不同字符的个数和每种字符出现的次数,按照升序排列输出。
相关问题
编写程序统计1行字符串中: 不同字符的个数。 每种字符出现的次数,升序输出字符
以下是 Python 代码实现:
```python
s = input("请输入一个字符串:")
char_count = {} # 用于存储每个字符出现的次数
# 统计每个字符出现的次数
for char in s:
if char not in char_count:
char_count[char] = 1
else:
char_count[char] += 1
# 输出不同字符的个数
print("不同字符的个数为:", len(char_count))
# 按照升序输出每种字符及其出现次数
for char in sorted(char_count.keys()):
print(char, char_count[char])
```
运行结果示例:
```
请输入一个字符串:hello world
不同字符的个数为: 9
1
d 1
e 1
h 1
l 3
o 2
r 1
w 1
```
解释说明:
首先输入一个字符串,例如 "hello world",然后创建一个空字典 `char_count` 用于存储每个字符出现的次数。
接着遍历字符串中的每个字符,如果该字符不在 `char_count` 中,则将其添加到 `char_count` 中,并设置出现次数为 1。否则,将该字符的出现次数加 1。
统计完所有字符出现的次数后,输出不同字符的个数,即 `char_count` 的长度。
最后按照升序输出每种字符及其出现次数,通过 `sorted(char_count.keys())` 将 `char_count` 中的键按照字典序升序排序,然后遍历每个键,输出该键及其对应的值。
用python编写程序统计1行字符串中: 不同字符的个数。 每种字符出现的次数。
可以使用字典来实现统计不同字符的个数和每种字符出现的次数。具体实现如下:
```python
s = input("请输入字符串:")
# 统计不同字符的个数
count = len(set(s))
print("不同字符的个数为:", count)
# 统计每种字符出现的次数
char_dict = {}
for c in s:
if c in char_dict:
char_dict[c] += 1
else:
char_dict[c] = 1
for k, v in char_dict.items():
print("字符 '{}' 出现了 {} 次".format(k, v))
```
其中,`set(s)` 可以得到字符串中所有不同的字符,`len(set(s))` 即可得到不同字符的个数。使用字典 `char_dict` 统计每种字符出现的次数,遍历字符串中的每个字符,如果字符已经在字典中,就将对应的值加 1,否则将该字符作为键添加到字典中,值为 1。最后遍历字典,输出每个键值对即可。
相关推荐
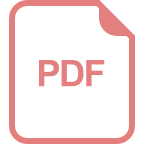
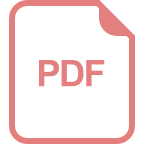
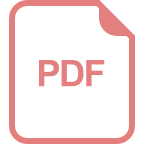
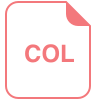
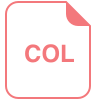
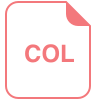
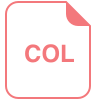
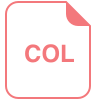







