#include<iostream> using namespace std; void swap(int &x,int &y) { int temp; temp=x; x=y; y=temp; } int main() { cout<<swap(3,5)<<endl; system("pause"); return 0; }这段代码的错误在哪
时间: 2023-12-03 12:01:58 浏览: 39
这段代码有两个错误:
1. `swap` 函数的返回类型应该是 `void`,因为它并不返回任何值。
2. `swap` 函数调用时传递的参数类型应该是变量的引用,而不是常量。因此,函数定义应该改为 `void swap(int& x, int& y)`,而调用应该改为 `swap(a, b)`,其中 `a` 和 `b` 是变量,而不是常量。
正确的代码应该是这样的:
```
#include<iostream>
using namespace std;
void swap(int& x, int& y) {
int temp;
temp = x;
x = y;
y = temp;
}
int main() {
int a = 3, b = 5;
swap(a, b);
cout << "a = " << a << ", b = " << b << endl;
system("pause");
return 0;
}
```
相关问题
#include <iostream> using namespace std; int a=10,b=20; int main(){ cout<<swap(a,b); return 0; }
你的代码中有一个小问题。C++ 中的 swap 函数没有返回值,因此在 `cout<<swap(a,b);` 这行代码中使用了一个无效的输出。如果你想输出交换后的值,你可以修改代码如下:
```cpp
#include <iostream>
using namespace std;
void swap(int& a, int& b) {
int temp = a;
a = b;
b = temp;
}
int main() {
int a = 10;
int b = 20;
swap(a, b);
cout << "a: " << a << endl;
cout << "b: " << b << endl;
return 0;
}
```
这段代码将会输出:
```
a: 20
b: 10
```
这样你就可以看到 `swap` 函数成功交换了 `a` 和 `b` 的值。请注意,我们在 `main` 函数中重新定义了 `a` 和 `b`,这样就不会与全局变量冲突。
下面代码哪里错了#include<iostream> #include<string> using namespace std; template<class T> void swap(T& A, T& B) { T temp = A; A = B; B = temp; } int main() { int A = 1; int B = 2; cout << "交换之前," << "A=" << A << "B=" << B << endl; swap(A, B); cout << "交换之前," << "A=" << A << "B=" << B << endl; return 0; }
代码有一个小错误,就是在输出交换之后的值时,输出的字符串中的"之前"应该改为"之后"。具体代码如下:
```c++
#include<iostream>
#include<string>
using namespace std;
template<class T>
void swap(T& A, T& B) {
T temp = A;
A = B;
B = temp;
}
int main() {
int A = 1;
int B = 2;
cout << "交换之前," << "A=" << A << "B=" << B << endl;
swap(A, B);
cout << "交换之后," << "A=" << A << "B=" << B << endl; //这里修改了输出字符串
return 0;
}
```
这个错误不会导致程序崩溃或出现其他异常行为,只是输出的结果不正确。
相关推荐
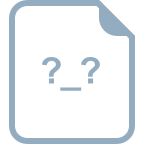












