Java批量上传图片到Linux服务器某个文件夹
时间: 2023-09-21 19:08:29 浏览: 104
可以使用Java中的JSch和SCP实现批量上传图片到Linux服务器某个文件夹。以下是一个示例代码:
```
import com.jcraft.jsch.*;
import java.io.File;
import java.io.FileInputStream;
import java.util.Properties;
public class UploadImages {
public static void main(String[] args) {
String localPath = "/path/to/local/folder"; // 本地图片文件夹路径
String remotePath = "/path/to/remote/folder"; // 远程Linux服务器文件夹路径
String username = "your_username"; // Linux服务器用户名
String password = "your_password"; // Linux服务器密码
String host = "your_linux_server_host"; // Linux服务器IP地址
JSch jsch = new JSch();
Session session = null;
Channel channel = null;
try {
session = jsch.getSession(username, host, 22);
session.setPassword(password);
Properties config = new Properties();
config.put("StrictHostKeyChecking", "no");
session.setConfig(config);
session.connect();
channel = session.openChannel("sftp");
channel.connect();
ChannelSftp sftp = (ChannelSftp) channel;
File localFolder = new File(localPath);
File[] files = localFolder.listFiles();
for (File file : files) {
if (file.isFile()) {
FileInputStream fis = new FileInputStream(file);
sftp.put(fis, remotePath + "/" + file.getName());
fis.close();
}
}
sftp.disconnect();
channel.disconnect();
session.disconnect();
System.out.println("上传完成");
} catch (JSchException | SftpException | java.io.IOException e) {
e.printStackTrace();
}
}
}
```
首先需要引入JSch的jar包,可以从官网下载。然后根据实际情况修改代码中的本地图片文件夹路径、远程Linux服务器文件夹路径、Linux服务器用户名、密码、IP地址等信息。运行代码后,程序会将本地图片文件夹中的所有图片上传到远程Linux服务器的指定文件夹中。
阅读全文
相关推荐
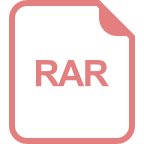
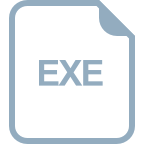
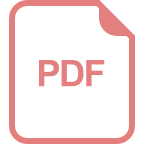
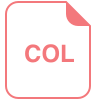
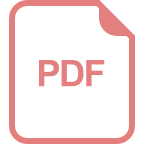
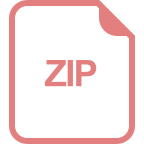
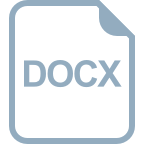
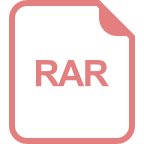
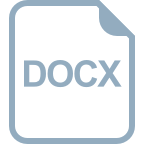
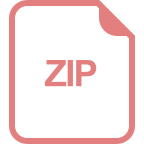
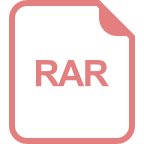
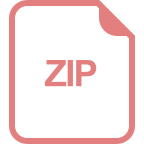
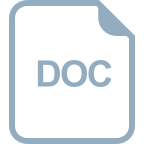
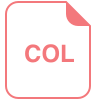
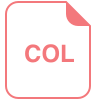