用python写一个简单的个人记账系统
时间: 2023-10-29 13:04:26 浏览: 65
好的,下面是一个简单的个人记账系统的Python实现,使用了Django框架:
首先,安装Django,可以使用以下命令:
```
pip install Django
```
然后,创建一个新的Django项目:
```
django-admin startproject account_book
```
接下来,创建一个新的Django应用程序:
```
cd account_book
python manage.py startapp transactions
```
在transactions应用程序中,创建一个名为"models.py"的文件,并定义以下模型:
```python
from django.db import models
class Category(models.Model):
name = models.CharField(max_length=100)
def __str__(self):
return self.name
class Transaction(models.Model):
description = models.CharField(max_length=200)
amount = models.DecimalField(max_digits=8, decimal_places=2)
date = models.DateField()
category = models.ForeignKey(Category, on_delete=models.CASCADE)
def __str__(self):
return self.description
```
在这个模型中,我们定义了两个模型类:Category和Transaction。Category用于表示交易类别,Transaction用于表示交易本身。Transaction模型包括一个外键,指向Category模型。
接下来,我们需要在"transactions/views.py"中定义视图函数:
```python
from django.shortcuts import render
from .models import Transaction
def transaction_list(request):
transactions = Transaction.objects.all()
return render(request, 'transactions/transaction_list.html', {'transactions': transactions})
```
在这个视图函数中,我们查询所有的交易,并将它们传递给模板。
接下来,我们需要创建一个模板来显示交易列表。在"transactions/templates/transactions/transaction_list.html"中,添加以下代码:
```html
{% extends 'base.html' %}
{% block content %}
<h1>Transaction List</h1>
<table>
<thead>
<tr>
<th>Description</th>
<th>Amount</th>
<th>Date</th>
<th>Category</th>
</tr>
</thead>
<tbody>
{% for transaction in transactions %}
<tr>
<td>{{ transaction.description }}</td>
<td>{{ transaction.amount }}</td>
<td>{{ transaction.date }}</td>
<td>{{ transaction.category }}</td>
</tr>
{% endfor %}
</tbody>
</table>
{% endblock %}
```
这个模板显示所有交易的列表,并包括每个交易的描述、金额、日期和类别。
最后,我们需要将这个视图函数添加到"transactions/urls.py"中,并将其包括在Django项目的主urls.py文件中:
```python
from django.urls import path
from .views import transaction_list
urlpatterns = [
path('', transaction_list, name='transaction_list'),
]
```
在主urls.py文件中,我们需要添加一个包括transactions应用程序urls.py文件的路径:
```python
from django.contrib import admin
from django.urls import path, include
urlpatterns = [
path('admin/', admin.site.urls),
path('transactions/', include('transactions.urls')),
]
```
现在,我们可以运行Django服务器并测试这个应用程序:
```
python manage.py runserver
```
现在,打开浏览器并访问"http://127.0.0.1:8000/transactions/",就可以看到交易列表了。
相关推荐
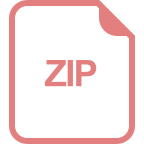
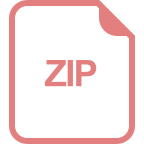
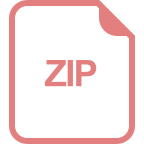












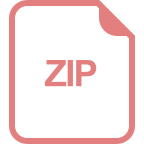
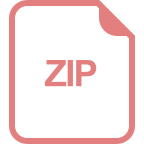
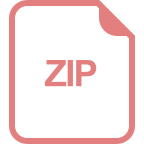