生成一个基于STM32的串口环形缓冲区代码
时间: 2024-03-16 09:47:30 浏览: 81
好的,以下是一个基于STM32的串口环形缓冲区代码示例:
```c
#define BUFFER_SIZE 256 // 缓冲区大小
uint8_t rx_buffer[BUFFER_SIZE]; // 接收缓冲区
volatile uint16_t rx_head = 0; // 接收缓冲区头指针
volatile uint16_t rx_tail = 0; // 接收缓冲区尾指针
uint8_t tx_buffer[BUFFER_SIZE]; // 发送缓冲区
volatile uint16_t tx_head = 0; // 发送缓冲区头指针
volatile uint16_t tx_tail = 0; // 发送缓冲区尾指针
void USART1_IRQHandler(void)
{
if (USART1->SR & USART_SR_RXNE) // 接收中断
{
uint8_t data = USART1->DR; // 读取接收到的数据
uint16_t next_head = (rx_head + 1) % BUFFER_SIZE; // 计算下一个头指针位置
if (next_head != rx_tail) // 判断缓冲区是否已满
{
rx_buffer[rx_head] = data; // 将数据写入缓冲区
rx_head = next_head; // 更新头指针位置
}
}
if (USART1->SR & USART_SR_TXE) // 发送中断
{
if (tx_head != tx_tail) // 判断缓冲区是否为空
{
uint8_t data = tx_buffer[tx_tail]; // 读取发送数据
USART1->DR = data; // 将数据发送出去
tx_tail = (tx_tail + 1) % BUFFER_SIZE; // 更新尾指针位置
}
else
{
USART1->CR1 &= ~USART_CR1_TXEIE; // 禁用发送中断
}
}
}
void usart1_send_data(uint8_t *data, uint16_t len)
{
uint16_t i;
for (i = 0; i < len; i++)
{
uint16_t next_head = (tx_head + 1) % BUFFER_SIZE; // 计算下一个头指针位置
while (next_head == tx_tail); // 等待缓冲区有空闲位置
tx_buffer[tx_head] = data[i]; // 将数据写入缓冲区
tx_head = next_head; // 更新头指针位置
USART1->CR1 |= USART_CR1_TXEIE; // 启用发送中断
}
}
uint16_t usart1_recv_data(uint8_t *data, uint16_t len)
{
uint16_t i;
for (i = 0; i < len; i++)
{
if (rx_head == rx_tail) // 判断缓冲区是否为空
{
break;
}
data[i] = rx_buffer[rx_tail]; // 读取数据
rx_tail = (rx_tail + 1) % BUFFER_SIZE; // 更新尾指针位置
}
return i;
}
```
这段代码实现了一个基于STM32的串口环形缓冲区,可以实现串口收发数据的缓存和处理。其中,接收缓冲区和发送缓冲区都是采用环形队列的方式实现,可以有效避免数据溢出和缺失的情况。同时,代码中也实现了发送和接收数据的函数接口,可以方便地进行使用。
阅读全文
相关推荐
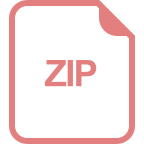
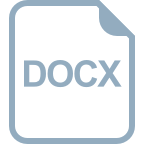
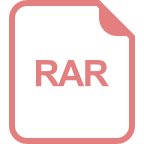
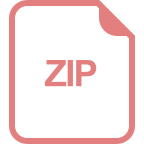
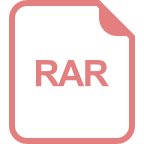
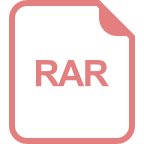
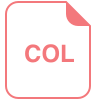
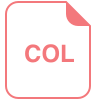
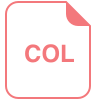
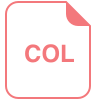
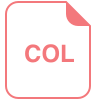
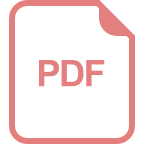
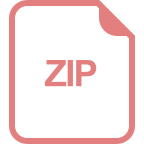