python利用八叉树划分三维点云代码
时间: 2023-10-21 14:19:23 浏览: 62
以下是Python使用八叉树划分三维点云的示例代码:
```python
import numpy as np
class OctreeNode:
def __init__(self, center, radius):
self.center = center
self.radius = radius
self.points = []
self.children = []
def insert(self, point):
if len(self.children) > 0:
index = self.get_child_index(point)
if index != -1:
self.children[index].insert(point)
return
self.points.append(point)
if len(self.points) > 8:
self.subdivide()
def subdivide(self):
radius = self.radius / 2
for i in range(8):
x = self.center[0] + radius * (-1)**(i%2)
y = self.center[1] + radius * (-1)**(i//2%2)
z = self.center[2] + radius * (-1)**(i//4)
child_center = np.array([x, y, z])
child_node = OctreeNode(child_center, radius)
for point in self.points:
child_node.insert(point)
self.children.append(child_node)
self.points = []
def get_child_index(self, point):
for i, child in enumerate(self.children):
if child.contains(point):
return i
return -1
def contains(self, point):
return np.linalg.norm(point - self.center) <= self.radius
def get_points_in_sphere(self, center, radius):
points = []
if not self.intersects_sphere(center, radius):
return points
for point in self.points:
if np.linalg.norm(point - center) <= radius:
points.append(point)
for child in self.children:
points.extend(child.get_points_in_sphere(center, radius))
return points
def intersects_sphere(self, center, radius):
return np.linalg.norm(center - self.center) <= self.radius + radius
class Octree:
def __init__(self, points, center, radius):
self.root = OctreeNode(center, radius)
for point in points:
self.root.insert(point)
def get_points_in_sphere(self, center, radius):
return self.root.get_points_in_sphere(center, radius)
# 示例使用
points = np.random.rand(1000, 3) * 10 - 5
octree = Octree(points, np.array([0, 0, 0]), 5)
points_in_sphere = octree.get_points_in_sphere(np.array([1, 2, 3]), 2)
print(len(points_in_sphere))
```
在此示例中,我们首先定义了一个`OctreeNode`类表示八叉树节点,其中`center`和`radius`属性表示节点的中心和半径。`points`属性是节点包含的点列表,而`children`属性则是子节点列表。`insert`方法将一个点插入节点,如果节点包含子节点,则将点插入适当的子节点中。如果节点包含的点数超过8,则将节点细分为8个子节点。`subdivide`方法创建8个子节点,并将原来节点包含的点分散到子节点中。`get_child_index`方法根据点的位置返回子节点的索引。`contains`方法检查节点是否包含给定的点。`get_points_in_sphere`方法返回在给定半径内的球体中的所有点的列表。`intersects_sphere`方法检查节点是否与球体相交。
`Octree`类使用`OctreeNode`类构建八叉树。在构造函数中,我们首先创建一个根节点,然后将所有点插入该节点。`get_points_in_sphere`方法使用根节点的`get_points_in_sphere`方法来查找在给定球体内的点。
在示例中,我们使用`numpy`生成1000个随机三维点,并使用它们创建一个八叉树。然后,我们使用`get_points_in_sphere`方法找到距离点`(1, 2, 3)`不超过2的所有点,并输出它们的数量。
相关推荐
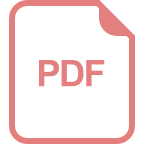














