#include <iostream> #include <vector> #include <string> using namespace std; class CDate{ public: CDate(); CDate (int year,int month,int day); CDate& operator ++(); CDate operator ++(int); CDate operator+(long days) const; CDate operator-(long days) const; long operator -(const CDate& rhs) const; int WeekDay() const; static int IsLeapYear(int year); friend ostream& operator <<(ostream& out,const CDate& d); friend istream& operator >>(istream& in, CDate& d); int getmonthday(); bool operator>(const CDate& d) const; private: int m_year,m_month,m_day; }; /* 请在这里填写答案 */ int main(int argc, char** argv) { vector<string> w={"SUN","MON","TUE","WEN","THU","FRI","SAT"}; CDate d1; int n; cin>>d1; cout<<d1<<endl; cout<<w[d1.WeekDay()]<<endl; cin>>n; CDate d2=d1+n; cout<<w[d2.WeekDay()]<<endl; ++d2; cout<<d2<<endl; d2++; cout<<d2<<endl; cout<<d2-d1<<endl; // cout<<d2-d1<<endl; return 0; }
时间: 2023-11-22 09:54:47 浏览: 95
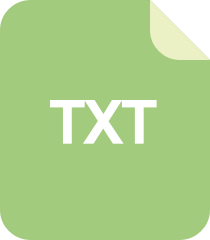
#include_iostream.h_

以下是填空部分的答案:
```c++
CDate::CDate() {
time_t t = time(NULL);
struct tm* now = localtime(&t);
m_year = now->tm_year + 1900;
m_month = now->tm_mon + 1;
m_day = now->tm_mday;
}
CDate::CDate(int year, int month, int day) : m_year(year), m_month(month), m_day(day) {}
CDate& CDate::operator++() {
if (m_day == getmonthday()) {
m_day = 1;
if (m_month == 12) {
m_year++;
m_month = 1;
} else {
m_month++;
}
} else {
m_day++;
}
return *this;
}
CDate CDate::operator++(int) {
CDate tmp(*this);
operator++();
return tmp;
}
CDate CDate::operator+(long days) const {
CDate tmp(*this);
if (days >= 0) {
for (long i = 0; i < days; i++) {
++tmp;
}
} else {
for (long i = 0; i > days; i--) {
--tmp;
}
}
return tmp;
}
CDate CDate::operator-(long days) const {
return operator+(-days);
}
long CDate::operator-(const CDate& rhs) const {
struct tm t1 = {0}, t2 = {0};
t1.tm_year = m_year - 1900;
t1.tm_mon = m_month - 1;
t1.tm_mday = m_day;
t2.tm_year = rhs.m_year - 1900;
t2.tm_mon = rhs.m_month - 1;
t2.tm_mday = rhs.m_day;
time_t time1 = mktime(&t1);
time_t time2 = mktime(&t2);
return (time1 - time2) / (24 * 60 * 60);
}
int CDate::WeekDay() const {
struct tm t = {0};
t.tm_year = m_year - 1900;
t.tm_mon = m_month - 1;
t.tm_mday = m_day;
mktime(&t);
return t.tm_wday;
}
int CDate::IsLeapYear(int year) {
if (year % 400 == 0 || (year % 4 == 0 && year % 100 != 0)) {
return 1;
} else {
return 0;
}
}
int CDate::getmonthday() {
int days[] = {31, 28 + IsLeapYear(m_year), 31, 30, 31, 30, 31, 31, 30, 31, 30, 31};
return days[m_month - 1];
}
bool CDate::operator>(const CDate& d) const {
if (m_year > d.m_year) {
return true;
} else if (m_year < d.m_year) {
return false;
} else {
if (m_month > d.m_month) {
return true;
} else if (m_month < d.m_month) {
return false;
} else {
if (m_day > d.m_day) {
return true;
} else {
return false;
}
}
}
}
ostream& operator<<(ostream& out, const CDate& d) {
out << d.m_year << "-" << d.m_month << "-" << d.m_day;
return out;
}
istream& operator>>(istream& in, CDate& d) {
in >> d.m_year >> d.m_month >> d.m_day;
return in;
}
```
阅读全文
相关推荐
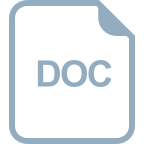
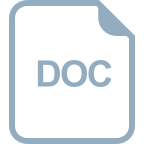
















