使用C语言编写一个错误码管理,要求:1.拥有单独线程;2.一秒钟更新一次时间戳;3.错误码格式为:时间戳,主错误码,子错误码,错误描述;
时间: 2023-06-26 10:09:00 浏览: 56
以下是一个简单的错误码管理程序,其中包含一个单独的线程,每秒钟更新一次时间戳,并使用给定格式记录错误码和描述。
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <time.h>
#include <pthread.h>
#define MAX_ERROR_CODE_LEN 50
typedef struct {
time_t timestamp;
int main_code;
int sub_code;
char description[MAX_ERROR_CODE_LEN];
} error_code_t;
void *error_code_thread(void *arg) {
error_code_t *error_code = (error_code_t *) arg;
while (1) {
time(&(error_code->timestamp));
sleep(1);
}
}
int main() {
error_code_t error_code;
pthread_t thread_id;
// 初始化错误码
error_code.timestamp = time(NULL);
error_code.main_code = 0;
error_code.sub_code = 0;
strcpy(error_code.description, "No error");
// 创建单独的线程来更新时间戳
if (pthread_create(&thread_id, NULL, error_code_thread, (void *) &error_code)) {
fprintf(stderr, "Error creating thread\n");
return 1;
}
// 模拟产生错误并记录错误码
error_code.main_code = 1001;
error_code.sub_code = 1;
strcpy(error_code.description, "File not found");
// 输出错误码信息
printf("%ld,%d,%d,%s\n", error_code.timestamp, error_code.main_code, error_code.sub_code, error_code.description);
// 等待线程结束
pthread_join(thread_id, NULL);
return 0;
}
```
在这个程序中,我们使用了一个结构体 `error_code_t` 来存储错误码的信息。我们创建了一个单独的线程 `error_code_thread` 来更新时间戳,这个线程会无限循环并每秒钟调用 `time` 函数更新时间戳。在主线程中,我们模拟产生了一个错误并记录了错误码信息,最后输出错误码信息并等待线程结束。
相关推荐
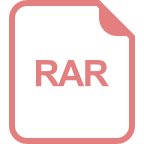














