在tiny c complier的基础上添加对变量声明语句的识别功能,并且说出相应的语法规则包括BNF和EBNF,并且说明其抽象语法树是如何设计的
时间: 2024-03-08 10:48:28 浏览: 27
在 Tiny C Compiler 的基础上添加变量声明语句的识别功能需要在语法分析器中添加相应的产生式。假设我们要添加的变量声明语句的语法规则是:
```
declaration ::= type identifier ;
type ::= int | float | double
```
其中,type 表示变量类型,identifier 表示变量名。这个语法规则的 BNF 形式如下:
```
<declaration> ::= <type> <identifier> ;
<type> ::= int | float | double
```
这个语法规则的 EBNF 形式如下:
```
<declaration> ::= <type> <identifier> ';'
<type> ::= 'int' | 'float' | 'double'
```
在抽象语法树中,变量声明语句可以表示为一个 Declaration 节点,其子节点包括 Type 节点和 Identifier 节点。其中 Type 节点表示变量类型,Identifier 节点表示变量名。代码示例:
```
typedef enum {
DECLARATION_NODE,
TYPE_NODE,
IDENTIFIER_NODE,
} NodeType;
typedef struct {
char *name; // 变量名
NodeType type; // 节点类型
struct Node *left; // Type 节点
struct Node *right; // Identifier 节点
} Node;
Node *new_declaration_node(Node *type_node, Node *identifier_node) {
Node *node = malloc(sizeof(Node));
node->type = DECLARATION_NODE;
node->left = type_node;
node->right = identifier_node;
return node;
}
Node *new_type_node(char *type_name) {
Node *node = malloc(sizeof(Node));
node->type = TYPE_NODE;
node->name = type_name;
return node;
}
Node *new_identifier_node(char *identifier_name) {
Node *node = malloc(sizeof(Node));
node->type = IDENTIFIER_NODE;
node->name = identifier_name;
return node;
}
```
在语法分析器中,可以通过递归下降法实现对变量声明语句的识别和抽象语法树的构造。代码示例:
```
// <declaration> ::= <type> <identifier> ';'
Node *declaration() {
Node *type_node = type();
Node *identifier_node = identifier();
match(TK_SEMICOLON);
return new_declaration_node(type_node, identifier_node);
}
// <type> ::= 'int' | 'float' | 'double'
Node *type() {
Token token = get_next_token();
if (token.type == TK_INT || token.type == TK_FLOAT || token.type == TK_DOUBLE) {
return new_type_node(token.value);
} else {
error("expect type");
return NULL;
}
}
// <identifier> ::= ID
Node *identifier() {
Token token = get_next_token();
if (token.type == TK_ID) {
return new_identifier_node(token.value);
} else {
error("expect identifier");
return NULL;
}
}
```
这样,我们就可以在 Tiny C Compiler 中添加变量声明语句的识别功能,并且使用抽象语法树来表示变量声明语句。
相关推荐
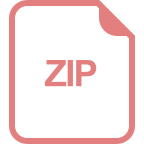














