C语言实现空间多边定位算法
时间: 2023-08-05 21:05:23 浏览: 145
空间多边定位算法主要是解决空间中多个定位点的坐标计算问题,它可以应用于定位系统、导航系统等领域。
下面是一个C语言实现空间多边定位算法的示例代码:
```c
#include <stdio.h>
#include <math.h>
#define PI 3.14159265358979323846
typedef struct {
double x;
double y;
double z;
} Point3D;
// 计算两个点之间的距离
double distance(Point3D p1, Point3D p2) {
return sqrt(pow(p1.x - p2.x, 2) + pow(p1.y - p2.y, 2) + pow(p1.z - p2.z, 2));
}
// 计算球面坐标
void get_spherical_coord(Point3D p, double *r, double *theta, double *phi) {
*r = sqrt(pow(p.x, 2) + pow(p.y, 2) + pow(p.z, 2));
*theta = atan2(p.y, p.x);
*phi = acos(p.z / (*r));
}
// 计算两个球面坐标之间的距离
double spherical_distance(double r1, double theta1, double phi1, double r2, double theta2, double phi2) {
return sqrt(pow(r1, 2) + pow(r2, 2) - 2 * r1 * r2 * (sin(phi1) * sin(phi2) * cos(theta1 - theta2) + cos(phi1) * cos(phi2)));
}
// 计算交点坐标
Point3D get_intersection(Point3D p1, Point3D p2, double r1, double r2) {
Point3D p;
double d = distance(p1, p2);
double a = (pow(r1, 2) - pow(r2, 2) + pow(d, 2)) / (2 * d);
double h = sqrt(pow(r1, 2) - pow(a, 2));
double x2 = p1.x + a * (p2.x - p1.x) / d;
double y2 = p1.y + a * (p2.y - p1.y) / d;
double z2 = p1.z + a * (p2.z - p1.z) / d;
p.x = x2 + h * (p2.z - p1.z) / d;
p.y = y2 - h * (p2.x - p1.x) / d;
p.z = z2 - h * (p2.y - p1.y) / d;
return p;
}
// 计算定位点坐标
Point3D locate(Point3D p1, Point3D p2, Point3D p3, double r1, double r2, double r3) {
double d1 = distance(p1, p2);
double d2 = distance(p2, p3);
double d3 = distance(p3, p1);
double theta1, phi1, theta2, phi2, theta3, phi3;
get_spherical_coord(p1, &r1, &theta1, &phi1);
get_spherical_coord(p2, &r2, &theta2, &phi2);
get_spherical_coord(p3, &r3, &theta3, &phi3);
Point3D i1 = get_intersection(p1, p2, r1, r2);
Point3D i2 = get_intersection(p2, p3, r2, r3);
Point3D i3 = get_intersection(p3, p1, r3, r1);
double d12 = spherical_distance(r1, theta1, phi1, r2, theta2, phi2);
double d23 = spherical_distance(r2, theta2, phi2, r3, theta3, phi3);
double d31 = spherical_distance(r3, theta3, phi3, r1, theta1, phi1);
double x = (i1.x * d23 + i2.x * d31 + i3.x * d12) / (d23 + d31 + d12);
double y = (i1.y * d23 + i2.y * d31 + i3.y * d12) / (d23 + d31 + d12);
double z = (i1.z * d23 + i2.z * d31 + i3.z * d12) / (d23 + d31 + d12);
Point3D p;
p.x = x;
p.y = y;
p.z = z;
return p;
}
int main() {
Point3D p1 = {0, 0, 0};
Point3D p2 = {0, 0, 1};
Point3D p3 = {1, 0, 0};
double r1 = 1;
double r2 = 1;
double r3 = 1;
Point3D p = locate(p1, p2, p3, r1, r2, r3);
printf("定位点坐标: (%lf, %lf, %lf)\n", p.x, p.y, p.z);
return 0;
}
```
在这个示例代码中,我们先定义了一个 `Point3D` 结构体,用来表示三维空间中的点。然后,我们实现了 `distance` 函数,用来计算两个点之间的距离。
接着,我们实现了 `get_spherical_coord` 函数,用来将一个点转换成球面坐标。我们还实现了 `spherical_distance` 函数,用来计算两个球面坐标之间的距离。
最后,我们实现了 `get_intersection` 函数,用来计算两个球体的交点坐标,以及 `locate` 函数,用来计算定位点坐标。
在 `main` 函数中,我们定义了三个定位点和它们的半径,然后调用 `locate` 函数计算定位点坐标,并输出结果。
阅读全文
相关推荐

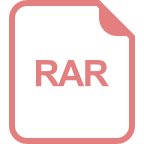
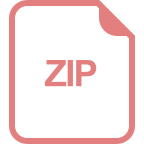
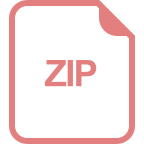
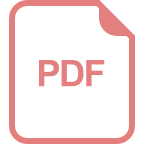
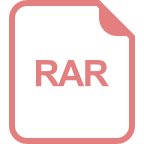
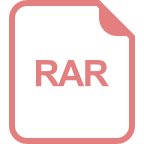
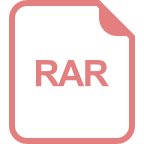
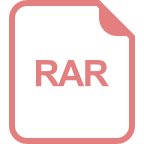
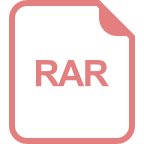
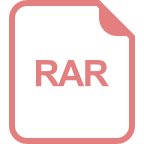
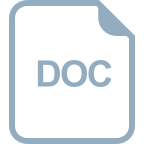
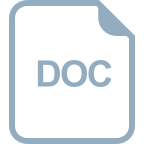
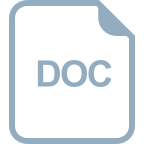
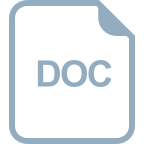
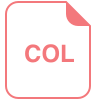