ava Swing 小项目:指针时钟 + 数字日期时钟 的实现
时间: 2024-02-05 08:14:29 浏览: 30
Java Swing 是 Java GUI 编程的重要工具包,可以用来开发桌面应用程序。下面是一个指针时钟和数字日期时钟的示例代码,代码中使用了 Java Swing 的一些常用组件和布局管理器:
```java
import java.awt.BorderLayout;
import java.awt.Color;
import java.awt.Font;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.text.SimpleDateFormat;
import java.util.Calendar;
import java.util.Date;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JPanel;
import javax.swing.Timer;
public class Clock extends JFrame {
private static final long serialVersionUID = 1L;
private JPanel panel;
private JLabel timeLabel;
private JLabel dateLabel;
private Timer timer;
public Clock() {
setTitle("Clock");
setSize(500, 300);
setDefaultCloseOperation(EXIT_ON_CLOSE);
setLocationRelativeTo(null);
panel = new JPanel(new BorderLayout());
timeLabel = new JLabel();
timeLabel.setFont(new Font("Arial", Font.BOLD, 60));
timeLabel.setForeground(Color.BLUE);
panel.add(timeLabel, BorderLayout.CENTER);
dateLabel = new JLabel();
dateLabel.setFont(new Font("Arial", Font.BOLD, 20));
dateLabel.setForeground(Color.BLACK);
panel.add(dateLabel, BorderLayout.SOUTH);
add(panel);
timer = new Timer(1000, new ActionListener() {
public void actionPerformed(ActionEvent e) {
updateTimeAndDate();
}
});
timer.start();
}
private void updateTimeAndDate() {
Date now = new Date();
String time = new SimpleDateFormat("HH:mm:ss").format(now);
String date = new SimpleDateFormat("yyyy-MM-dd").format(now);
timeLabel.setText(time);
dateLabel.setText(date);
}
public static void main(String[] args) {
Clock clock = new Clock();
clock.setVisible(true);
}
}
```
上面的示例代码中,我们创建了一个 `Clock` 类,继承自 `JFrame`,并且实现了一个指针时钟和数字日期时钟的功能。在构造方法中,我们创建了一个 `JPanel`,用于放置时钟和日期标签。时钟标签使用了 `JLabel` 组件,并设置了字体和颜色,日期标签与之类似。
我们使用了 `Timer` 来实现每秒钟更新时钟和日期的操作。在 `updateTimeAndDate()` 方法中,我们获取当前时间和日期,并将其格式化为特定的字符串格式,然后更新时钟和日期标签的文本。
最后,在 `main()` 方法中,我们创建了一个 `Clock` 对象,并设置其可见性。运行程序后,我们可以看到一个漂亮的指针时钟和数字日期时钟。
相关推荐
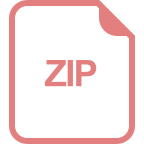
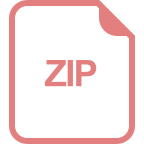
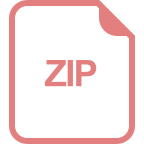














