Unity PC与蓝牙通信
时间: 2024-05-03 15:23:19 浏览: 11
Unity可以使用C#脚本来实现与蓝牙设备的通信。首先需要使用蓝牙设备的SDK或API来连接蓝牙设备,并获取设备的ID或地址。
在Unity中,可以使用C#的SerialPort类来实现串口通信,因为蓝牙设备在计算机上被识别为串口设备。以下是一个示例代码,用于连接蓝牙设备并发送数据:
```csharp
using System.IO.Ports;
SerialPort bluetooth = new SerialPort("COM1", 9600); // Replace COM1 with your Bluetooth device port and 9600 with your device's baud rate
bluetooth.Open(); // Open the port
if (bluetooth.IsOpen) // Check if the port is open
{
bluetooth.Write("Hello, World!"); // Send data to the device
}
bluetooth.Close(); // Close the port
```
请注意,此示例代码将COM1用作蓝牙设备端口。您需要将其替换为您的蓝牙设备端口。还需要将9600替换为您的设备的波特率。
为了接收数据,您可以在SerialPort对象上注册一个DataReceived事件,然后在事件处理程序中处理数据。以下是一个示例代码:
```csharp
using System.IO.Ports;
SerialPort bluetooth = new SerialPort("COM1", 9600); // Replace COM1 with your Bluetooth device port and 9600 with your device's baud rate
bluetooth.DataReceived += Bluetooth_DataReceived; // Register the DataReceived event
void Bluetooth_DataReceived(object sender, SerialDataReceivedEventArgs e)
{
string data = bluetooth.ReadLine(); // Read the incoming data
Debug.Log(data); // Output the data to the console
}
bluetooth.Open(); // Open the port
```
请注意,这里使用了ReadLine()方法来读取接收到的数据。如果您的蓝牙设备使用不同的数据格式,请相应地更改代码。
总的来说,使用C#的SerialPort类与蓝牙设备通信是相对简单的,并且可以在Unity中轻松实现。
相关推荐
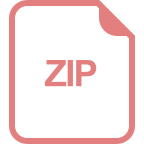














