用Java写一个窗口化的图书管理系统
时间: 2023-08-22 15:09:59 浏览: 74
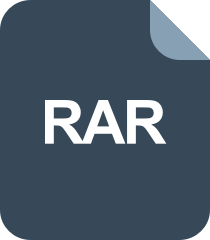
用java写的一个图书管理系统

好的,下面是一个简单的Java图书管理系统的代码示例:
```java
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.util.ArrayList;
public class BookManagementSystem extends JFrame implements ActionListener {
private JLabel titleLabel, authorLabel, priceLabel, searchLabel;
private JTextField titleField, authorField, priceField, searchField;
private JButton addButton, searchButton, deleteButton;
private JTable bookTable;
private JScrollPane scrollPane;
private ArrayList<Book> bookList;
// 构造函数
public BookManagementSystem() {
setTitle("图书管理系统");
setSize(600, 400);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
// 创建组件
titleLabel = new JLabel("书名:");
authorLabel = new JLabel("作者:");
priceLabel = new JLabel("价格:");
searchLabel = new JLabel("搜索:");
titleField = new JTextField();
authorField = new JTextField();
priceField = new JTextField();
searchField = new JTextField();
addButton = new JButton("添加");
searchButton = new JButton("搜索");
deleteButton = new JButton("删除");
bookList = new ArrayList<>();
bookTable = new JTable(new BookTableModel(bookList));
scrollPane = new JScrollPane(bookTable);
// 设置布局
setLayout(new GridBagLayout());
GridBagConstraints c = new GridBagConstraints();
// 添加书名、作者、价格标签和文本框
c.gridx = 0;
c.gridy = 0;
c.insets = new Insets(10, 10, 10, 10);
add(titleLabel, c);
c.gridx = 1;
add(titleField, c);
c.gridx = 0;
c.gridy = 1;
add(authorLabel, c);
c.gridx = 1;
add(authorField, c);
c.gridx = 0;
c.gridy = 2;
add(priceLabel, c);
c.gridx = 1;
add(priceField, c);
// 添加按钮
c.gridx = 0;
c.gridy = 3;
add(addButton, c);
c.gridx = 1;
add(deleteButton, c);
c.gridx = 2;
add(searchLabel, c);
c.gridx = 3;
add(searchField, c);
c.gridx = 4;
add(searchButton, c);
// 添加书籍列表
c.gridx = 0;
c.gridy = 4;
c.gridwidth = 5;
c.fill = GridBagConstraints.BOTH; // 填充整个空间
c.weightx = 1.0; // 水平扩展
c.weighty = 1.0; // 垂直扩展
add(scrollPane, c);
// 添加事件监听器
addButton.addActionListener(this);
deleteButton.addActionListener(this);
searchButton.addActionListener(this);
}
// 添加书籍
private void addBook() {
String title = titleField.getText();
String author = authorField.getText();
double price = Double.parseDouble(priceField.getText());
Book book = new Book(title, author, price);
bookList.add(book);
// 刷新表格
bookTable.setModel(new BookTableModel(bookList));
// 清空文本框
titleField.setText("");
authorField.setText("");
priceField.setText("");
}
// 删除书籍
private void deleteBook() {
int row = bookTable.getSelectedRow();
if (row >= 0) {
bookList.remove(row);
bookTable.setModel(new BookTableModel(bookList));
}
}
// 搜索书籍
private void searchBook() {
String keyword = searchField.getText();
ArrayList<Book> result = new ArrayList<>();
for (Book book : bookList) {
if (book.getTitle().contains(keyword) || book.getAuthor().contains(keyword)) {
result.add(book);
}
}
// 刷新表格
bookTable.setModel(new BookTableModel(result));
}
// 事件处理
@Override
public void actionPerformed(ActionEvent e) {
if (e.getSource() == addButton) {
addBook();
} else if (e.getSource() == deleteButton) {
deleteBook();
} else if (e.getSource() == searchButton) {
searchBook();
}
}
public static void main(String[] args) {
BookManagementSystem system = new BookManagementSystem();
system.setVisible(true);
}
}
// 书籍类
class Book {
private String title;
private String author;
private double price;
public Book(String title, String author, double price) {
this.title = title;
this.author = author;
this.price = price;
}
public String getTitle() {
return title;
}
public String getAuthor() {
return author;
}
public double getPrice() {
return price;
}
}
// 表格模型类
class BookTableModel extends AbstractTableModel {
private ArrayList<Book> bookList;
private String[] columnNames = {"书名", "作者", "价格"};
public BookTableModel(ArrayList<Book> bookList) {
this.bookList = bookList;
}
@Override
public int getRowCount() {
return bookList.size();
}
@Override
public int getColumnCount() {
return 3;
}
@Override
public String getColumnName(int column) {
return columnNames[column];
}
@Override
public Object getValueAt(int rowIndex, int columnIndex) {
Book book = bookList.get(rowIndex);
switch (columnIndex) {
case 0:
return book.getTitle();
case 1:
return book.getAuthor();
case 2:
return book.getPrice();
default:
return null;
}
}
}
```
这个图书管理系统包括添加、删除和搜索书籍的功能,以及一个表格来显示所有的书籍。界面使用了Java的Swing库来实现。
阅读全文
相关推荐

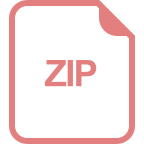



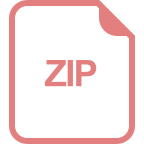
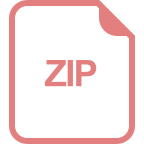
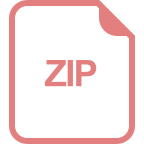
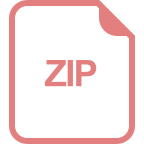
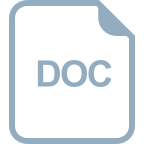

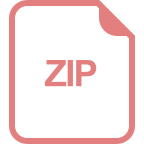
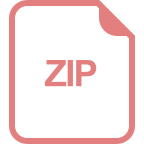