使用vue2写一段评论回复功能代码
时间: 2023-08-30 20:06:21 浏览: 48
好的,下面是一个简单的评论回复功能的Vue组件代码示例:
```vue
<template>
<div class="comment">
<div class="comment-list">
<div v-for="comment in comments" :key="comment.id" class="comment-item">
<div class="comment-info">
<span class="comment-author">{{ comment.author }}</span>
<span class="comment-time">{{ comment.time }}</span>
</div>
<div class="comment-content">{{ comment.content }}</div>
<div class="comment-reply">
<a href="#" @click="showReplyForm(comment.id)">回复</a>
</div>
<div class="comment-reply-form" v-show="replyCommentId === comment.id">
<textarea v-model="replyContent"></textarea>
<button @click="submitReply(comment.id)">提交</button>
</div>
<div class="comment-replies">
<div v-for="reply in comment.replies" :key="reply.id" class="comment-reply-item">
<div class="comment-info">
<span class="comment-author">{{ reply.author }}</span>
<span class="comment-time">{{ reply.time }}</span>
</div>
<div class="comment-content">{{ reply.content }}</div>
</div>
</div>
</div>
</div>
<div class="comment-form">
<textarea v-model="newCommentContent"></textarea>
<button @click="submitComment">提交</button>
</div>
</div>
</template>
<script>
export default {
data() {
return {
comments: [
{
id: 1,
author: '张三',
time: '2021-08-01 10:00:00',
content: '这是一条评论',
replies: [],
},
{
id: 2,
author: '李四',
time: '2021-08-02 11:00:00',
content: '这是另一条评论',
replies: [],
},
],
newCommentContent: '',
replyCommentId: null,
replyContent: '',
};
},
methods: {
showReplyForm(commentId) {
this.replyCommentId = commentId;
},
submitReply(commentId) {
const comment = this.comments.find((c) => c.id === commentId);
if (comment) {
comment.replies.push({
id: Date.now(),
author: '匿名用户',
time: new Date().toLocaleString(),
content: this.replyContent,
});
this.replyContent = '';
this.replyCommentId = null;
}
},
submitComment() {
this.comments.push({
id: Date.now(),
author: '匿名用户',
time: new Date().toLocaleString(),
content: this.newCommentContent,
replies: [],
});
this.newCommentContent = '';
},
},
};
</script>
```
在上述代码中,我们使用了Vue的响应式数据来存储评论数据,包括评论内容、评论时间、作者等信息。同时,我们还使用了`v-for`指令来循环渲染评论列表和回复列表。当用户点击“回复”按钮时,会显示回复表单,用户填写完回复内容后,点击“提交”按钮即可提交回复。当用户在评论区域填写完评论内容后,点击“提交”按钮即可提交评论。需要注意的是,在实际开发过程中,还需要考虑到数据校验、安全性等问题。
相关推荐











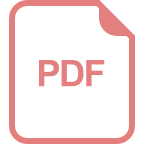