tensorflow实现1维CNN-LSTM多头自注意力机制
时间: 2023-10-19 09:05:43 浏览: 50
下面是一个基于TensorFlow实现的1维CNN-LSTM多头自注意力机制的示例代码:
```python
import tensorflow as tf
from tensorflow.keras import layers
class MultiHeadSelfAttention(layers.Layer):
def __init__(self, embed_dim, num_heads):
super(MultiHeadSelfAttention, self).__init__()
self.embed_dim = embed_dim
self.num_heads = num_heads
self.head_dim = embed_dim // num_heads
assert self.head_dim * num_heads == embed_dim, "Embedding dimension must be divisible by number of heads."
self.query_dense = layers.Dense(embed_dim)
self.key_dense = layers.Dense(embed_dim)
self.value_dense = layers.Dense(embed_dim)
self.combine_heads = layers.Dense(embed_dim)
def attention(self, query, key, value):
score = tf.matmul(query, key, transpose_b=True)
dim_scaled_score = score / tf.math.sqrt(tf.cast(self.head_dim, dtype=tf.float32))
attention_weights = tf.nn.softmax(dim_scaled_score, axis=-1)
attention_output = tf.matmul(attention_weights, value)
return attention_output, attention_weights
def split_heads(self, x, batch_size):
x = tf.reshape(x, [batch_size, -1, self.num_heads, self.head_dim])
return tf.transpose(x, perm=[0, 2, 1, 3])
def call(self, inputs):
batch_size = tf.shape(inputs)[0]
query = self.query_dense(inputs)
key = self.key_dense(inputs)
value = self.value_dense(inputs)
query = self.split_heads(query, batch_size)
key = self.split_heads(key, batch_size)
value = self.split_heads(value, batch_size)
attention_output, _ = self.attention(query, key, value)
attention_output = tf.transpose(attention_output, perm=[0, 2, 1, 3])
concat_attention = tf.reshape(attention_output, [batch_size, -1, self.embed_dim])
output = self.combine_heads(concat_attention)
return output
class CNN_LSTM_MultiHeadAttention(tf.keras.Model):
def __init__(self, num_classes, num_heads, dropout_rate):
super(CNN_LSTM_MultiHeadAttention, self).__init__()
self.conv1d = layers.Conv1D(filters=128, kernel_size=3, padding='same', activation='relu')
self.pooling = layers.MaxPooling1D(pool_size=2, strides=2)
self.lstm = layers.LSTM(units=64, return_sequences=True)
self.dropout = layers.Dropout(dropout_rate)
self.attention = MultiHeadSelfAttention(embed_dim=64, num_heads=num_heads)
self.flatten = layers.Flatten()
self.dense = layers.Dense(num_classes, activation='softmax')
def call(self, inputs):
x = self.conv1d(inputs)
x = self.pooling(x)
x = self.lstm(x)
x = self.dropout(x)
x = self.attention(x)
x = self.flatten(x)
output = self.dense(x)
return output
```
上面的代码中,`MultiHeadSelfAttention`类实现了多头自注意力机制,`CNN_LSTM_MultiHeadAttention`类则使用了1维CNN、LSTM和多头自注意力机制来构建模型。其中,`num_classes`指定了分类的类别数,`num_heads`指定了注意力机制中注意头的数量,`dropout_rate`指定了dropout的比例。在`call`方法中,输入数据首先经过1维卷积层和池化层,然后经过LSTM层和dropout层,接着经过多头自注意力机制,最后通过全连接层输出分类结果。
该模型可以通过如下代码进行编译和训练:
```python
model = CNN_LSTM_MultiHeadAttention(num_classes=10, num_heads=8, dropout_rate=0.2)
model.compile(optimizer='adam', loss='categorical_crossentropy', metrics=['accuracy'])
model.fit(x_train, y_train, batch_size=32, epochs=10, validation_data=(x_val, y_val))
```
其中,`x_train`和`y_train`是训练数据,`x_val`和`y_val`是验证数据。在训练过程中,采用了Adam优化器和交叉熵损失函数。
相关推荐
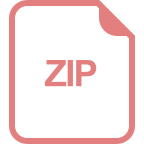
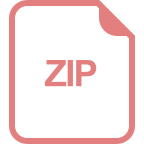
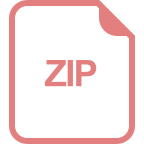














