springaop最简单的应用
时间: 2023-10-28 13:03:35 浏览: 49
Spring AOP(面向切面编程)是Spring框架提供的一个重要特性,用于在运行时动态地将代码切入到类的指定方法、指定位置进行增强。
下面是Spring AOP最简单的应用示例:
1. 首先,在pom.xml文件中添加以下依赖:
```xml
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-aop</artifactId>
<version>5.1.9.RELEASE</version>
</dependency>
```
2. 创建一个接口和它的实现类:
```java
public interface HelloService {
void sayHello(String name);
}
public class HelloServiceImpl implements HelloService {
@Override
public void sayHello(String name) {
System.out.println("Hello, " + name);
}
}
```
3. 创建一个切面类,在方法执行前后打印日志:
```java
@Aspect
public class LogAspect {
@Before("execution(* com.example.service.HelloService.*(..))")
public void before(JoinPoint joinPoint) {
System.out.println("Before method: " + joinPoint.getSignature().getName());
}
@After("execution(* com.example.service.HelloService.*(..))")
public void after(JoinPoint joinPoint) {
System.out.println("After method: " + joinPoint.getSignature().getName());
}
}
```
4. 在Spring配置文件中配置:
```xml
<bean id="helloService" class="com.example.service.HelloServiceImpl"></bean>
<bean id="logAspect" class="com.example.aspect.LogAspect"></bean>
<aop:config>
<aop:aspect ref="logAspect">
<aop:pointcut id="helloMethod" expression="execution(* com.example.service.HelloService.*(..))"/>
<aop:before pointcut-ref="helloMethod" method="before"/>
<aop:after pointcut-ref="helloMethod" method="after"/>
</aop:aspect>
</aop:config>
```
5. 测试:
```java
public class Main {
public static void main(String[] args) {
ApplicationContext context = new ClassPathXmlApplicationContext("applicationContext.xml");
HelloService helloService = (HelloService)context.getBean("helloService");
helloService.sayHello("John");
}
}
```
输出结果:
```
Before method: sayHello
Hello, John
After method: sayHello
```
以上就是Spring AOP最简单的应用示例。通过这个示例,我们可以看到如何在Spring中使用AOP来实现切面编程。
相关推荐
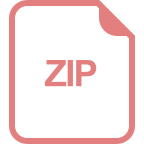
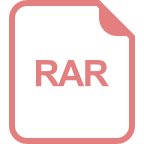
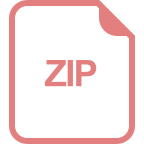














