sjz.html:1 Uncaught TypeError: Failed to resolve module specifier "three". Import Map: "three" matches with "three" but is blocked by a null value 错误怎么处理
时间: 2024-03-02 19:49:59 浏览: 27
这个错误的意思是在你的代码中使用了模块导入,但是模块的路径无法被解析。具体来说,这个错误是因为你使用了一个名为 "three" 的模块,但是它没有被正确加载或安装。
可能的解决方案是:
1. 确保你已经安装了 "three" 模块。你可以在终端或命令行中使用命令 `npm install three` 或 `yarn add three` 来安装它。
2. 如果你已经安装了 "three" 模块,检查你的代码中是否正确导入了它。你可以使用相对路径或绝对路径导入模块,例如 `import * as THREE from './path/to/three.js'` 或 `import * as THREE from '/absolute/path/to/three.js'`。
3. 如果你正在使用导入映射(Import Map)来管理模块路径,确保你已经正确配置了映射。你可以在 HTML 文件的 `<script type="importmap">` 标签中定义映射,例如:
```html
<script type="importmap">
{
"imports": {
"three": "./path/to/three.js"
}
}
</script>
```
这个映射将 "three" 模块映射到了 "./path/to/three.js" 文件。注意,这个特性还不是所有浏览器都支持,所以你可能需要在使用之前检查一下浏览器的兼容性。
相关推荐
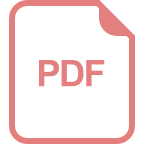
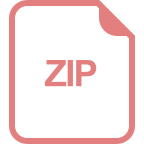
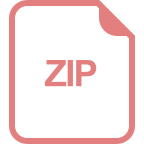
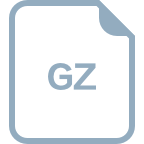
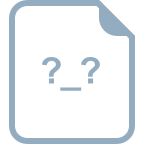
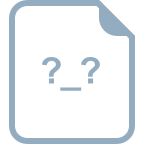
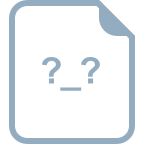