2.编程统计候选人的得票数。设有3个候选人zhang、li、wang(候选 人姓名不区分大小写),10个选民,选民每次输入一个候选人的名字,若 选民输错候选人的姓名,则按废票处理。选民投票结束后,程序自动显 示各候选人的得票结果和废票数要求利用结构体数组表示3个候选人 的姓名和得票结果,将最终的统计结果显宗输出并写入文本文件中。
时间: 2024-02-25 17:52:25 浏览: 18
以下是一个简单的C++程序,可以实现统计候选人得票数的功能,同时将结果输出到控制台和文本文件中:
```
#include <iostream>
#include <fstream>
#include <string>
using namespace std;
struct Candidate {
string name;
int votes;
};
int main() {
Candidate candidates[3] = {{"zhang", 0}, {"li", 0}, {"wang", 0}};
int invalid_votes = 0;
for (int i = 0; i < 10; i++) {
string name;
cout << "Please enter the name of the candidate (zhang/li/wang): ";
cin >> name;
if (name == "zhang" || name == "Zhang") {
candidates[0].votes++;
} else if (name == "li" || name == "Li") {
candidates[1].votes++;
} else if (name == "wang" || name == "Wang") {
candidates[2].votes++;
} else {
invalid_votes++;
}
}
// 输出结果到控制台
cout << "Candidate\tVotes" << endl;
for (int i = 0; i < 3; i++) {
cout << candidates[i].name << "\t\t" << candidates[i].votes << endl;
}
cout << "Invalid votes: " << invalid_votes << endl;
// 输出结果到文本文件
ofstream outfile("result.txt");
outfile << "Candidate\tVotes" << endl;
for (int i = 0; i < 3; i++) {
outfile << candidates[i].name << "\t\t" << candidates[i].votes << endl;
}
outfile << "Invalid votes: " << invalid_votes << endl;
outfile.close();
return 0;
}
```
程序通过一个 `Candidate` 结构体数组来存储3个候选人的姓名和得票数,每次循环中根据选民输入的候选人名字来更新候选人的得票数。如果选民输入的名字不是 `zhang`、`li` 或 `wang`,则将其视为废票。循环结束后,程序将统计结果输出到控制台和文本文件中。
注意,在实际应用中,我们应该加入一些容错处理,比如对选民输入的名字进行大小写不敏感的比较,或者限制选民最多只能输入一定次数的错误名字等。
相关推荐
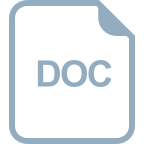



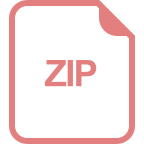
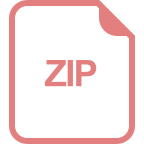