C语言链表插入节点
时间: 2023-06-28 20:04:18 浏览: 138
以下是一份简单的C语言代码,用于在链表中插入新的节点:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构体
struct Node {
int data;
struct Node* next;
};
// 插入节点函数
void insertNode(struct Node** head_ref, int new_data) {
// 创建新节点
struct Node* new_node = (struct Node*)malloc(sizeof(struct Node));
// 将新节点的数据设置为传入的参数
new_node->data = new_data;
// 将新节点的下一个节点指向头节点
new_node->next = (*head_ref);
// 将头节点指向新节点
(*head_ref) = new_node;
}
// 打印链表函数
void printList(struct Node* node) {
while (node != NULL) {
printf("%d ", node->data);
node = node->next;
}
}
int main() {
// 初始化空链表
struct Node* head = NULL;
// 插入节点
insertNode(&head, 1);
insertNode(&head, 2);
insertNode(&head, 3);
// 打印链表
printList(head);
return 0;
}
```
在上面的代码中,我们定义了一个结构体 `Node` 来表示链表中的每个节点。我们还定义了两个函数: `insertNode` 和 `printList`。
`insertNode` 函数用于在链表中插入新的节点。它接受一个指向头节点的指针 `head_ref` 和一个新节点的数据 `new_data`。首先,我们创建一个新的节点并为其分配内存。然后,我们将新节点的数据设置为 `new_data`。接下来,我们将新节点的下一个节点指向头节点,并将头节点指向新节点。
`printList` 函数用于遍历链表并打印每个节点的数据。它接受一个指向头节点的指针 `node`。我们使用一个 `while` 循环遍历链表,直到 `node` 等于 `NULL`(即链表的末尾)。在每个节点上,我们打印节点的数据并将 `node` 指向下一个节点。
在 `main` 函数中,我们首先初始化一个空链表。然后,我们使用 `insertNode` 函数插入三个节点。最后,我们使用 `printList` 函数打印链表中的所有节点的数据。
这就是一个简单的链表插入节点的C语言代码。
阅读全文
相关推荐
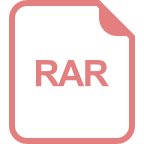
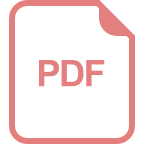
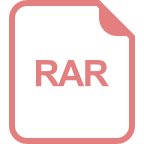
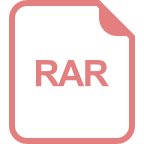
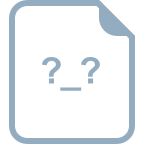
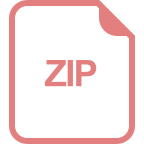
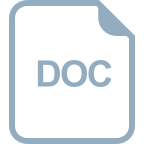
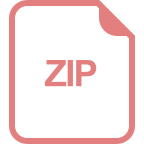
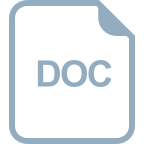
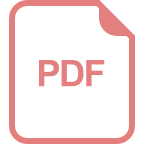
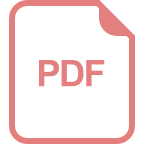
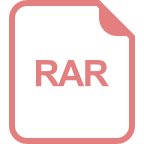
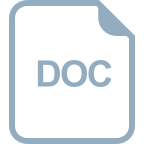
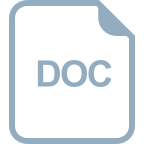

