写出在jupyter notebook中将分词好的文本数据进行停用词去除的程序
时间: 2023-03-11 14:35:47 浏览: 87
可以使用以下程序实现:# 加载停用词
stopwords = open("stopwords.txt", "r", encoding="utf-8").readlines()
stopwords = [word.strip() for word in stopwords]# 定义函数,将分词好的文本数据去除停用词
def remove_stopwords(text):
words = [w for w in text if w not in stopwords]
return words
相关问题
写出关于以下要求的程序,要求在jupyter notebook中将分词好的文本数据进行停用词去除
我可以为你提供一个简单的程序,可以在jupyter notebook中将分词好的文本数据进行停用词去除。首先,你需要导入必要的库,包括nltk和pandas。其次,你需要下载停用词表,并将它们存入一个变量中。然后,你需要读取分词好的文本数据,并将它们存入另一个变量中。最后,你可以使用循环将文本中的停用词去除,然后将新的文本存入另一个变量中。
写出关于以下要求的程序,要求在jupyter notebook中将分词好的一列进行停用词去除
### 回答1:
我可以为您提供一个简单的示例代码,可以帮助您在Jupyter notebook中去除分词好的一列中的停用词:import nltk
from nltk.corpus import stopwords# 读取已经分词好的列
words = []# 初始化停用词列表
stop_words = set(stopwords.words('english'))# 对文本列表进行停用词过滤
filtered_words = [w for w in words if not w in stop_words]
### 回答2:
在Jupyter Notebook中进行停用词去除的程序可以按照以下步骤实现:
1. 导入所需要的库:
```python
import jieba
```
2. 定义停用词列表,将需要去除的停用词添加到其中。停用词可以是中文常见的虚词或无实际意义的词语。
```python
stop_words = ['的', '了', '在', '是', '我', '你']
```
3. 定义一个方法用于去除停用词:
```python
def remove_stopwords(text):
seg_list = jieba.cut(text) # 使用jieba进行分词
filtered_words = []
for word in seg_list:
if word not in stop_words:
filtered_words.append(word)
return ' '.join(filtered_words) # 返回去除停用词的文本
```
4. 读取待处理的文本文件,并将分词结果保存在一个新的文件中:
```python
with open('input.txt', 'r', encoding='utf-8') as f:
text = f.read()
filtered_text = remove_stopwords(text)
with open('output.txt', 'w', encoding='utf-8') as outfile:
outfile.write(filtered_text)
```
在这个程序中,我们首先导入了jieba库用于分词。然后定义了停用词列表stop_words,并使用jieba.cut方法对待处理的文本进行分词。然后遍历分词结果,将不在停用词列表中的词语添加到filtered_words中。最后使用' '.join方法将过滤后的词语重新组合成去除停用词的文本。最后,将输出结果保存在一个新的文件中。
### 回答3:
在 Jupyter Notebook 中进行分词和停用词去除的程序可以使用 Python 的第三方库进行实现。以下是一个示例程序:
1. 首先,需要安装和导入所需的库:
```python
!pip install jieba # 安装分词库
!pip install nltk # 安装自然语言处理库
import jieba # 导入分词库
from nltk.corpus import stopwords # 导入停用词库
from nltk.tokenize import word_tokenize # 导入分词函数
```
2. 定义停用词列表,这些词将在分词后被去除:
```python
stopwords = stopwords.words('chinese') # 获取中文停用词列表
```
3. 定义分词和停用词去除函数 `remove_stopwords`:
```python
def remove_stopwords(text):
words = word_tokenize(text) # 分词
# 去除停用词
words = [word for word in words if word not in stopwords]
return words
```
4. 读取需要处理的文本文件并进行分词和停用词去除:
```python
# 读取文本文件并进行处理
with open('input.txt', 'r', encoding='utf-8') as file:
text = file.read() # 读取文本内容
words = remove_stopwords(text) # 分词和停用词去除
# 输出处理结果
print(words)
```
以上所示程序假设需要处理的文本文件名为 "input.txt",文本内容以 UTF-8 编码保存。程序将会打印出经分词和停用词去除后的结果。
请注意,在运行程序之前,需要安装相应的库以及准备好待处理的文本文件。
相关推荐
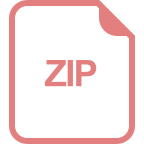












