php对应附件的全文检索如何实现?
时间: 2024-03-26 13:36:10 浏览: 95
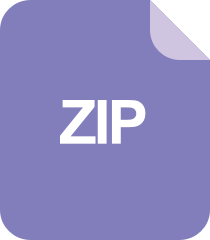
Attachment 实现 全文检索
PHP 对应附件的全文检索可以使用专门的全文检索引擎,如 Elasticsearch 等。以下是一个使用 Elasticsearch 实现对应附件的全文检索的示例代码:
1. 准备数据
假设我们有一个文章表 `articles`,其中有一列 `attachments` 存储了文章的附件信息,包括附件的文件名和内容。我们需要将这些附件内容索引到 Elasticsearch 中,以便后续进行全文检索。
```php
// 连接数据库
$conn = mysqli_connect('localhost', 'username', 'password', 'database');
// 查询所有文章
$query = "SELECT * FROM articles";
$result = mysqli_query($conn, $query);
// 创建 Elasticsearch 客户端
$client = Elasticsearch\ClientBuilder::create()->build();
// 循环遍历文章
while ($row = mysqli_fetch_assoc($result)) {
// 获取文章附件信息
$attachments = json_decode($row['attachments'], true);
// 循环遍历附件
foreach ($attachments as $attachment) {
// 判断附件是否已经索引过
$params = [
'index' => 'attachments',
'body' => [
'query' => [
'term' => [
'filename' => $attachment['filename']
]
]
]
];
$response = $client->search($params);
if ($response['hits']['total']['value'] > 0) {
continue;
}
// 将附件内容索引到 Elasticsearch 中
$params = [
'index' => 'attachments',
'body' => [
'filename' => $attachment['filename'],
'content' => $attachment['content']
]
];
$client->index($params);
}
}
```
2. 进行全文检索
当需要进行全文检索时,我们可以通过 Elasticsearch 的 `multi_match` 查询来实现对文章和附件的全文检索。
```php
// 创建 Elasticsearch 客户端
$client = Elasticsearch\ClientBuilder::create()->build();
// 查询语句
$params = [
'index' => ['articles', 'attachments'],
'body' => [
'query' => [
'multi_match' => [
'query' => 'keyword',
'fields' => ['title', 'content', 'filename', 'attachment_content']
]
]
]
];
// 执行查询
$response = $client->search($params);
// 处理查询结果
foreach ($response['hits']['hits'] as $hit) {
// 输出结果
}
```
以上是一个使用 Elasticsearch 实现对应附件的全文检索的示例代码,具体实现可以根据实际需求和使用场景进行调整。
阅读全文
相关推荐
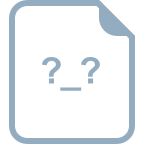
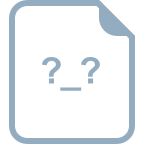
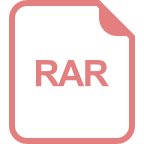
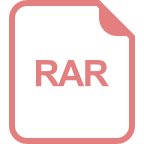
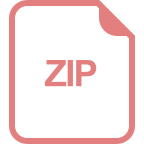
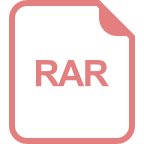
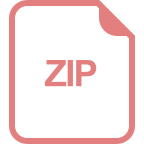
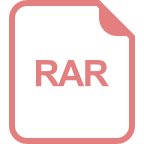
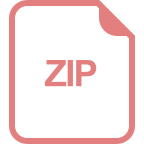
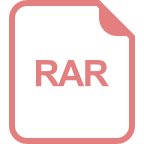
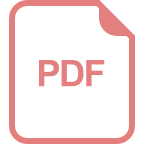
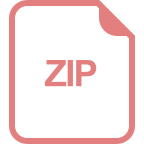
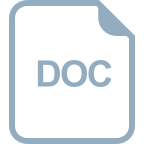


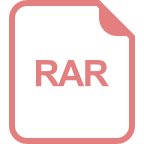