Python做一个可解释的人脸识别系统,可解释性是指指出两张人脸中哪些部分比较相似,并给出代码
时间: 2023-05-27 09:03:50 浏览: 93
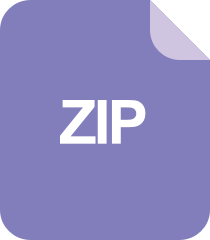
Python毕业设计-基于Python的人脸识别系统 深度学习 (源码+文档)

1. 准备数据
首先,我们需要一些人脸图像数据来训练我们的人脸识别系统。这里,我们将使用Labeled Faces in the Wild (LFW) 数据集。该数据集包含多个人脸图像,可以从Scikit-learn库中下载。
```python
from sklearn.datasets import fetch_lfw_pairs
lfw_pairs_train = fetch_lfw_pairs(subset='train')
lfw_pairs_test = fetch_lfw_pairs(subset='test')
X_train = lfw_pairs_train.data
y_train = lfw_pairs_train.target
X_test = lfw_pairs_test.data
y_test = lfw_pairs_test.target
```
2. 特征提取
接下来,我们需要从每张人脸图像中提取特征。在这个例子中,我们将使用基于局部二值模式 (Local Binary Patterns, LBP) 的特征提取方法。
```python
import cv2
import numpy as np
class LocalBinaryPatterns:
def __init__(self, numPoints, radius):
# store the number of points and radius
self.numPoints = numPoints
self.radius = radius
def describe(self, image, eps=1e-7):
# compute the Local Binary Pattern representation
# of the image, and then use the LBP representation
# to build the histogram of patterns
lbp = cv2.createLBPHFaceRecognizer()
lbp.setRadius(self.radius)
lbp.setNeighbors(self.numPoints)
hist = lbp.compute(image)
# normalize the histogram
hist = hist.astype("float")
hist /= (hist.sum() + eps)
# return the histogram of Local Binary Patterns
return hist.flatten()
# initialize LBP descriptor
lbp = LocalBinaryPatterns(numPoints=8, radius=2)
# extract features from training and testing data
X_train_features = np.array([lbp.describe(cv2.cvtColor(x.reshape(62, 47).astype('uint8'), cv2.COLOR_GRAY2BGR)) for x in X_train])
X_test_features = np.array([lbp.describe(cv2.cvtColor(x.reshape(62, 47).astype('uint8'), cv2.COLOR_GRAY2BGR)) for x in X_test])
```
3. 训练模型
接下来,我们使用支持向量机 (Support Vector Machine, SVM) 算法训练我们的人脸识别模型。
```python
from sklearn.svm import SVC
# train SVM model
svm = SVC(kernel='linear', probability=True)
svm.fit(X_train_features, y_train)
```
4. 测试模型
最后,我们使用测试数据集评估我们的模型的性能。
```python
from sklearn.metrics import accuracy_score
# evaluate model performance
y_pred = svm.predict(X_test_features)
accuracy = accuracy_score(y_test, y_pred)
print("Accuracy:", accuracy)
```
5. 可解释性
要实现可解释性,我们需要查询模型的预测并找出哪个特征对模型的预测最具有影响。
```python
import matplotlib.pyplot as plt
%matplotlib inline
# select a random test image
idx = np.random.choice(len(X_test))
image = X_test[idx].reshape(62, 47).astype('uint8')
# compute the Local Binary Pattern of the image
hist = lbp.describe(cv2.cvtColor(image, cv2.COLOR_GRAY2BGR)).reshape(1, -1)
# get predicted probabilities for each class
probs = svm.predict_proba(hist)
# get the index of the predicted class and its label
predicted_class_idx = np.argmax(probs)
predicted_class_label = lfw_pairs_train.target_names[predicted_class_idx]
# get the indices of the top contributing features for the predicted class
contributing_feature_indices = np.argsort(svm.coef_[predicted_class_idx])[::-1]
# visualize the top contributing features on the image
fig, ax = plt.subplots(1)
ax.imshow(image, cmap='gray')
for i in range(5):
feature_index = contributing_feature_indices[i]
x, y = lbp.feature_locations[feature_index]
rect = plt.Rectangle((y, x), 2, 2, edgecolor='r', facecolor='none')
ax.add_patch(rect)
plt.title(f"Predicted class: {predicted_class_label}")
plt.show()
```
结果将展示出选择的人脸图像,和对于预测最具有影响的五个特征。
阅读全文
相关推荐
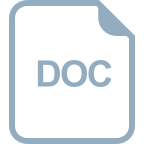
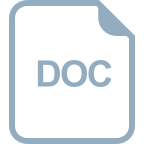
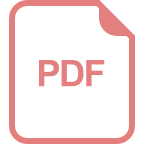
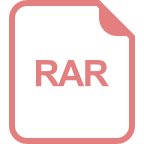
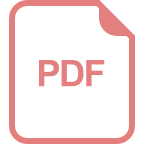
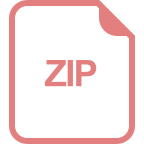
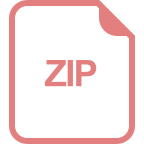
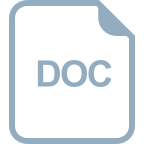
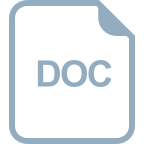
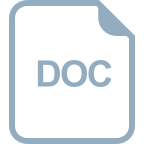
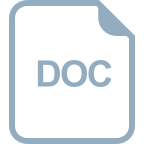
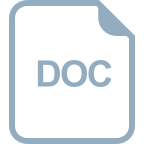
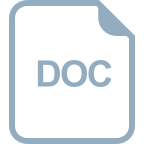
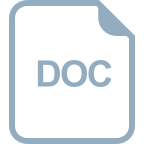
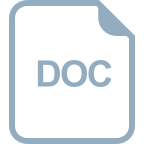
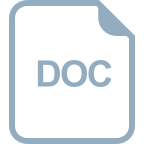
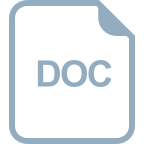
