python提取excel中列名为电压、电流、时间的数据
时间: 2024-04-09 17:33:58 浏览: 106
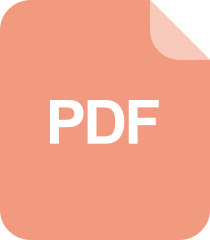
python读取excel数据
要提取Excel中特定列名为"电压"、"电流"和"时间"的数据,您可以使用`pandas`库。以下是一个示例代码:
```python
import pandas as pd
# 替换为您的Excel文件路径
excel_file = '/path/to/excel_file.xlsx'
# 读取Excel文件
df = pd.read_excel(excel_file)
# 提取特定列的数据
voltage_data = df['电压']
current_data = df['电流']
time_data = df['时间']
# 打印提取到的数据
print("电压数据:")
print(voltage_data)
print("\n电流数据:")
print(current_data)
print("\n时间数据:")
print(time_data)
```
请确保已经安装了`pandas`库,您可以使用`pip install pandas`命令进行安装。在上述代码中,我们使用`pd.read_excel`函数读取Excel文件,并将所需列名作为索引来提取相应的数据。最后,我们将每列的数据打印出来。
阅读全文
相关推荐
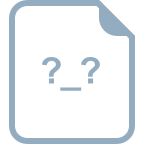
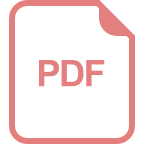
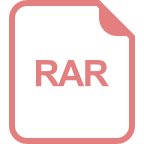
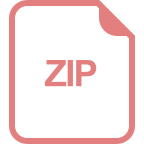
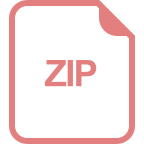
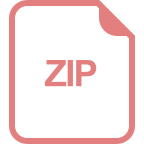
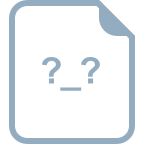
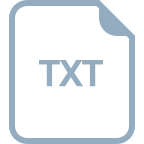
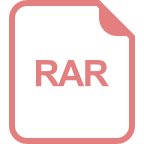
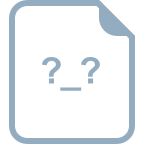
