vhdl实现uart程序
时间: 2023-07-30 18:06:41 浏览: 155
下面是一个简单的 VHDL 代码实现 UART 的例子程序:
```
library IEEE;
use IEEE.STD_LOGIC_1164.ALL;
use IEEE.NUMERIC_STD.ALL;
entity UART_Test is
Port ( CLK: in std_logic;
RST: in std_logic;
RX: in std_logic;
TX: out std_logic);
end UART_Test;
architecture Behavioral of UART_Test is
signal TX_busy : std_logic := '0';
signal TX_data : std_logic_vector(7 downto 0);
signal TX_counter : integer range 0 to 11 := 0;
signal RX_data : std_logic_vector(7 downto 0);
signal RX_counter : integer range 0 to 11 := 0;
signal RX_busy : std_logic := '0';
signal RX_done : std_logic := '0';
constant BAUD_RATE : integer := 9600;
constant CLK_FREQ : integer := 50000000; -- 50 MHz clock
begin
UART_TX : process(CLK, RST)
begin
if(RST = '1') then
TX_busy <= '0';
TX_data <= (others => '0');
TX_counter <= 0;
elsif(CLK'event and CLK = '1') then
if(TX_busy = '1') then
TX_counter <= TX_counter + 1;
if(TX_counter = 11) then
TX_data <= (others => '0');
TX_busy <= '0';
TX_counter <= 0;
end if;
else
if(RX_done = '1') then
TX_data <= RX_data;
TX_busy <= '1';
RX_done <= '0';
TX_counter <= 0;
end if;
end if;
end if;
end process UART_TX;
UART_RX : process(CLK, RST)
begin
if(RST = '1') then
RX_busy <= '0';
RX_data <= (others => '0');
RX_counter <= 0;
elsif(CLK'event and CLK = '1') then
if(RX_busy = '1') then
RX_counter <= RX_counter + 1;
if(RX_counter = 11) then
RX_done <= '1';
RX_busy <= '0';
RX_counter <= 0;
end if;
else
if(RX = '0') then
RX_busy <= '1';
RX_counter <= 0;
end if;
end if;
end if;
end process UART_RX;
-- Baud rate generator
UART_BAUD : process(CLK, RST)
variable baud_counter : integer range 0 to round(CLK_FREQ / (BAUD_RATE * 16)) - 1;
begin
if(RST = '1') then
baud_counter := 0;
elsif(CLK'event and CLK = '1') then
baud_counter := baud_counter + 1;
if(baud_counter = round(CLK_FREQ / (BAUD_RATE * 16)) - 1) then
baud_counter := 0;
end if;
end if;
if(baud_counter = 0) then
TX <= TX_data(TX_data'high);
TX_data <= TX_data(TX_data'high - 1 downto 0) & '0';
else
TX <= '1';
end if;
end process UART_BAUD;
end Behavioral;
```
该程序包括了一个简单的波特率生成器和 TX 和 RX 的状态机。在该程序中,当接收到数据后,它会被发送回去。该实现使用9600波特率,50 MHz时钟频率和8个数据位。为了测试该程序,你可以将一个串口转USB适配器连接到计算机上,然后将TX和RX引脚连接到适配器的TX和RX引脚上,就可以在计算机上使用串口工具来测试UART程序了。
阅读全文
相关推荐

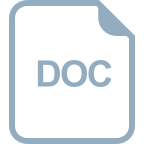
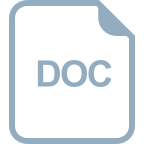
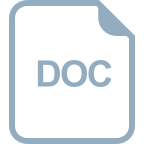
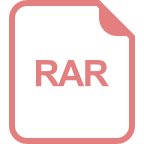
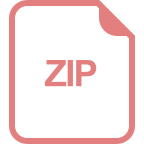
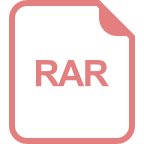
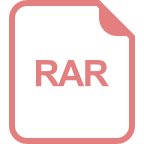
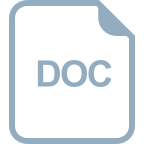
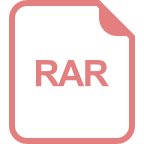
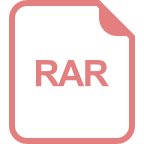
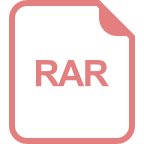
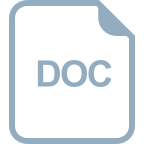
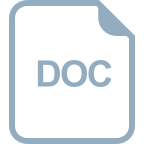
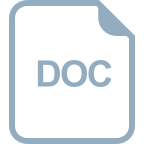
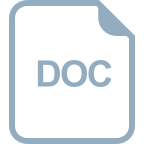