o写一个代码实现利用麦轮跑坐标点进行微调
时间: 2023-12-03 22:44:46 浏览: 74
这里提供一个简单的Python代码示例,利用麦轮小车跑坐标点进行微调:
```python
import math
import time
from ev3dev2.motor import LargeMotor, OUTPUT_B, OUTPUT_C
from ev3dev2.sensor import INPUT_1, INPUT_2
from ev3dev2.sensor.lego import ColorSensor, TouchSensor
# 初始化马达和传感器对象
left_motor = LargeMotor(OUTPUT_B)
right_motor = LargeMotor(OUTPUT_C)
color_sensor = ColorSensor(INPUT_1)
touch_sensor = TouchSensor(INPUT_2)
# 定义麦轮小车的参数
wheel_diameter = 5.6 # cm
wheel_circumference = wheel_diameter * math.pi # cm
wheel_distance = 12.0 # cm
# 定义目标坐标点列表
points = [(10, 10), (20, 10), (20, 20), (10, 20), (10, 10)]
for point in points:
target_x, target_y = point
print("Moving to point ({}, {})".format(target_x, target_y))
# 初始化当前位置和角度
current_x, current_y = 0, 0
current_angle = 0
# 计算目标点与当前位置的距离和角度
delta_x = target_x - current_x
delta_y = target_y - current_y
target_distance = math.sqrt(delta_x ** 2 + delta_y ** 2)
target_angle = math.atan2(delta_y, delta_x) - current_angle
if target_angle > math.pi:
target_angle -= 2 * math.pi
elif target_angle < -math.pi:
target_angle += 2 * math.pi
# 转向目标角度
while abs(target_angle) > 0.1:
if target_angle > 0:
left_motor.run_forever(speed_sp=100)
right_motor.run_forever(speed_sp=-100)
else:
left_motor.run_forever(speed_sp=-100)
right_motor.run_forever(speed_sp=100)
current_angle += color_sensor.reflected_light_intensity * 0.01
if current_angle > math.pi:
current_angle -= 2 * math.pi
elif current_angle < -math.pi:
current_angle += 2 * math.pi
target_angle = math.atan2(delta_y, delta_x) - current_angle
if target_angle > math.pi:
target_angle -= 2 * math.pi
elif target_angle < -math.pi:
target_angle += 2 * math.pi
# 前进到目标点
while target_distance > 0:
speed = target_distance / 5 + 20
left_motor.run_forever(speed_sp=speed)
right_motor.run_forever(speed_sp=speed)
current_x += math.cos(current_angle) * wheel_circumference * color_sensor.reflected_light_intensity / 100
current_y += math.sin(current_angle) * wheel_circumference * color_sensor.reflected_light_intensity / 100
target_distance = math.sqrt((target_x - current_x) ** 2 + (target_y - current_y) ** 2)
# 到达目标点后停止马达
left_motor.stop()
right_motor.stop()
# 稍作停顿,等待麦轮小车稳定
time.sleep(1)
```
这个代码会依次移动到预设的目标点列表中的每一个点,利用麦轮小车的传感器进行微调。在实际应用中,需要根据不同的场景进行调整,例如:调整马达速度、传感器灵敏度、停顿时间等参数。
阅读全文
相关推荐
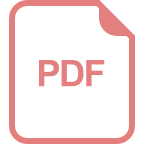
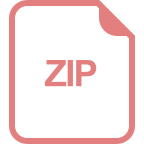
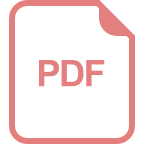
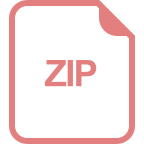
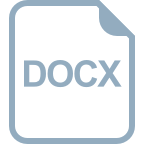
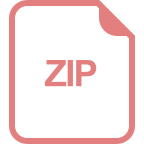
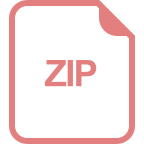
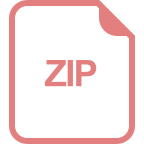
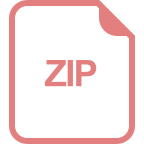
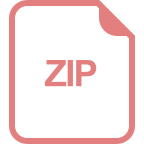
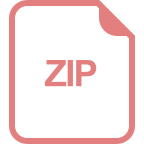
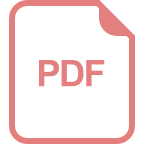
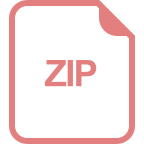
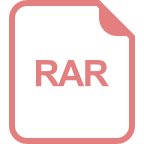
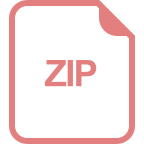
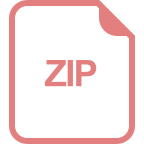