javascript花店代码程序
时间: 2023-07-03 11:02:48 浏览: 120
### 回答1:
JavaScript花店代码程序是一个用于模拟花店销售和管理的程序。该程序使用JavaScript编写,可以在网页浏览器上运行。
程序主要包括以下功能:
1. 花束展示:程序会在网页上展示花店的花束和价格。通过数组或对象的方式存储花束的名称和价格,并通过循环遍历将花束信息展示在网页上。
2. 购买花束:用户可以通过点击购买按钮来购买花束。购买花束时,程序会检查用户输入的花束数量是否合法,并计算出购买花束的总价。
3. 购物车:程序使用一个数组或对象来存储用户购买的花束信息。当用户点击购买按钮时,程序会将购买的花束信息添加到购物车中。用户可以在购物车中查看已购买的花束和总价。
4. 订单生成:当用户确认购物车中的订单后,程序会生成一个新的订单,并将订单信息存储到一个数组或对象中。订单信息包括订单号、购买的花束、总价等。
5. 订单管理:程序可以展示订单列表,包括订单号、购买的花束、总价等信息。用户可以选择查看特定订单的详细信息,或者删除已完成的订单。
6. 在线支付:用户可以选择在线支付购买的花束。程序会跳转到支付平台,让用户输入付款信息,完成支付流程。
7. 数据存储:程序使用本地存储或数据库来保存花束、订单和用户信息。这样可以确保用户的购物信息在关闭网页后不会丢失。
通过以上功能,JavaScript花店代码程序可以实现花店的销售和管理需求。用户可以方便地浏览和购买花束,管理订单信息,并完成安全的在线支付。
### 回答2:
花店的JavaScript代码程序可以用来实现以下功能:
1. 显示花店的库存列表,包括花卉的名称、描述和价格。
2. 添加新的花卉到库存中,包括花卉的名称、描述和价格。
3. 根据花卉的名称搜索库存中的花卉,并显示其详细信息。
4. 根据价格范围搜索库存中的花卉,并显示符合条件的花卉列表。
5. 更新花卉的价格。
6. 删除库存中的花卉。
以下是一个简单的花店JavaScript代码的示例:
// 花卉对象构造函数
function Flower(name, description, price) {
this.name = name;
this.description = description;
this.price = price;
}
// 创建花卉库存数组
var flowerInventory = [];
// 添加花卉到库存中
function addFlowerToInventory(name, description, price) {
var flower = new Flower(name, description, price);
flowerInventory.push(flower);
}
// 显示库存列表
function displayInventory() {
for (var i = 0; i < flowerInventory.length; i++) {
console.log("名称:" + flowerInventory[i].name);
console.log("描述:" + flowerInventory[i].description);
console.log("价格:" + flowerInventory[i].price);
console.log("-----------------------------");
}
}
//搜索花卉并显示详细信息
function searchFlowerByName(name) {
for (var i = 0; i < flowerInventory.length; i++) {
if (flowerInventory[i].name === name) {
console.log("名称:" + flowerInventory[i].name);
console.log("描述:" + flowerInventory[i].description);
console.log("价格:" + flowerInventory[i].price);
console.log("-----------------------------");
return;
}
}
console.log("找不到名称为" + name + "的花卉。");
}
// 根据价格范围搜索花卉并显示列表
function searchFlowerByPriceRange(minPrice, maxPrice) {
var foundFlowers = [];
for (var i = 0; i < flowerInventory.length; i++) {
if (flowerInventory[i].price >= minPrice && flowerInventory[i].price <= maxPrice) {
foundFlowers.push(flowerInventory[i]);
}
}
if (foundFlowers.length > 0) {
for (var j = 0; j < foundFlowers.length; j++) {
console.log("名称:" + foundFlowers[j].name);
console.log("描述:" + foundFlowers[j].description);
console.log("价格:" + foundFlowers[j].price);
console.log("-----------------------------");
}
} else {
console.log("找不到符合价格范围的花卉。");
}
}
// 更新花卉的价格
function updateFlowerPrice(name, newPrice) {
for (var i = 0; i < flowerInventory.length; i++) {
if (flowerInventory[i].name === name) {
flowerInventory[i].price = newPrice;
console.log("花卉价格已更新。");
return;
}
}
console.log("找不到名称为" + name + "的花卉。");
}
// 从库存中删除花卉
function deleteFlowerFromInventory(name) {
for (var i = 0; i < flowerInventory.length; i++) {
if (flowerInventory[i].name === name) {
flowerInventory.splice(i, 1);
console.log("花卉已从库存中删除。");
return;
}
}
console.log("找不到名称为" + name + "的花卉。");
}
// 测试代码
addFlowerToInventory("玫瑰", "这是一束美丽的红玫瑰", 20);
addFlowerToInventory("郁金香", "这是一束迷人的紫色郁金香", 15);
addFlowerToInventory("百合", "这是一朵纯洁的白色百合", 25);
displayInventory();
searchFlowerByName("郁金香");
searchFlowerByPriceRange(10, 22);
updateFlowerPrice("玫瑰", 25);
deleteFlowerFromInventory("百合");
阅读全文
相关推荐
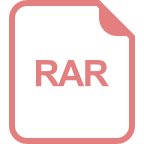
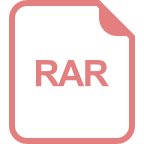
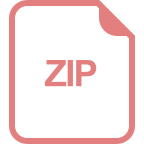
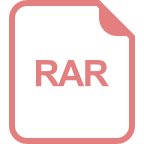
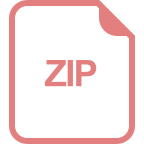
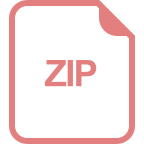
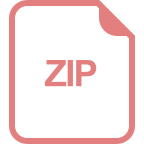
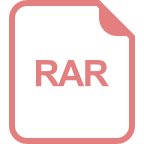
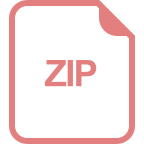
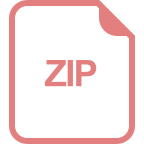
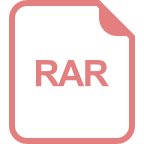
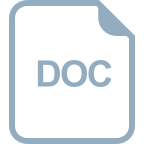
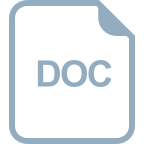
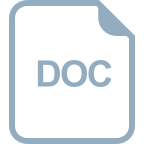
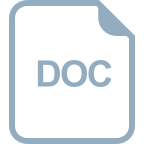
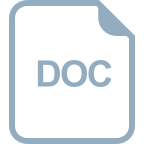