java 对接国标摄像头流程、代码整合 springboot SIP -GB2818
时间: 2024-03-17 16:44:39 浏览: 214
对接国标摄像头需要使用 SIP 协议进行通信,以下是整合 springboot 和 SIP 协议的代码示例:
1. 添加依赖
在 pom.xml 文件中添加以下依赖:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-sip</artifactId>
<version>2.4.5</version>
</dependency>
```
2. 配置 SIP
在 application.properties 文件中添加以下配置:
```properties
# SIP server configuration
spring.sip.server.address=192.168.0.1
spring.sip.server.port=5060
spring.sip.server.transport=udp
# SIP client configuration
spring.sip.client.address=192.168.0.2
spring.sip.client.port=5060
spring.sip.client.transport=udp
spring.sip.client.username=username
spring.sip.client.password=password
```
3. 编写代码
创建一个 SIP 消息监听器,用于处理 SIP 消息:
```java
@Component
public class SipListener implements MessageListener {
@Autowired
private SipSessionRegistry sipSessionRegistry;
@Override
public void onMessage(SipMessage sipMessage) {
// 处理 SIP 消息
}
}
```
创建一个 SIP 客户端,用于向 SIP 服务器发送请求:
```java
@Service
public class SipClient {
@Autowired
private SipSessionRegistry sipSessionRegistry;
@Autowired
private SipMessageFactory sipMessageFactory;
@Autowired
private SipClientTransactionFactory sipClientTransactionFactory;
@Autowired
private SipServerLocator sipServerLocator;
public void sendInvite(String address) throws Exception {
Request request = sipMessageFactory.createRequest(
"INVITE", "sip:" + address + ":5060", "SIP/2.0");
// 添加必要的头部信息
request.addHeader("From", "<sip:username@192.168.0.2>;tag=123456");
request.addHeader("To", "<sip:" + address + ":5060>");
request.addHeader("Call-ID", UUID.randomUUID().toString());
request.addHeader("CSeq", "1 INVITE");
request.addHeader("Contact", "<sip:username@192.168.0.2>");
request.addHeader("Content-Type", "application/sdp");
// 设置 SDP
String sdp = "v=0\r\n" +
"o=- 0 0 IN IP4 192.168.0.2\r\n" +
"s=Java SIP Client\r\n" +
"c=IN IP4 192.168.0.2\r\n" +
"t=0 0\r\n" +
"m=video 5004 RTP/AVP 96\r\n" +
"a=rtpmap:96 H264/90000\r\n" +
"a=fmtp:96 packetization-mode=1; profile-level-id=42001E; sprop-parameter-sets=Z00AH5pmDoAtyA==,aOvssiw=\r\n" +
"a=sendonly";
request.setContent(sdp, "application/sdp");
// 发送 INVITE 请求
SipClientTransaction transaction = sipClientTransactionFactory.createClientTransaction(request);
transaction.sendRequest();
// 将会话保存到会话注册表中
SipSession session = new SipSession();
session.setTransaction(transaction);
session.setRemoteAddress(address);
session.setRemotePort(5060);
sipSessionRegistry.addSession(session);
}
}
```
4. 调用 SIP 客户端
在需要发送 INVITE 请求的地方调用 SIP 客户端的 sendInvite 方法即可:
```java
@Autowired
private SipClient sipClient;
public void sendInviteRequest(String address) throws Exception {
sipClient.sendInvite(address);
}
```
5. 配置国标摄像头
配置国标摄像头需要使用国标协议,以下是配置国标摄像头的代码示例:
```java
public void configureCamera(String address) throws Exception {
// 创建 SIP 客户端
SipClient sipClient = new SipClient();
// 发送 INVITE 请求
sipClient.sendInvite(address);
// 等待响应
Thread.sleep(5000);
// 获取 SDP
SipSession session = sipSessionRegistry.getSession(address);
String sdp = session.getResponse().getContent().toString();
// 解析 SDP 获取视频流地址
String videoUrl = parseVideoUrlFromSdp(sdp);
// 配置国标摄像头
configureGbCamera(videoUrl);
}
```
以上就是整合 springboot 和 SIP 协议进行国标摄像头对接的流程和代码示例。
阅读全文
相关推荐
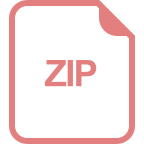
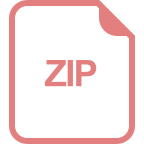
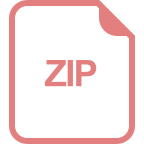
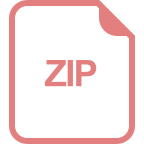
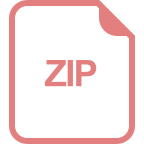
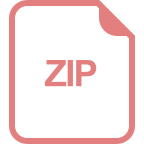
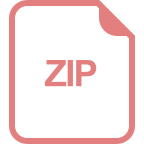
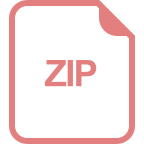
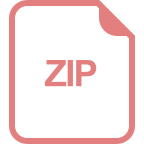
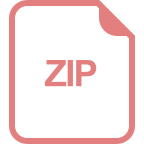
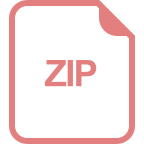
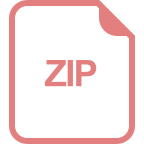
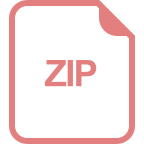
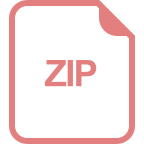
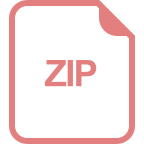
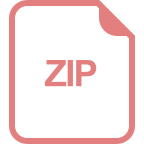
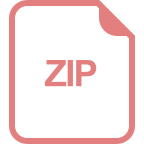