c#窗体应用程序实现当软件开启,定时器就在数据库扫描是否有设置的良品和不良品条码的当天ICT测试记录(ICT_test表中Barcode,Date,Result),良品和不良品条码在本地文件夹txt文件中获取。如果数据库中有良品和不良品测试记录存在,lable状态栏变绿并提示“已点检,请进行测试”,然后进行别的条码的ICT测试。条码先扫描到c#窗体软件txt_barcode中,在通过模拟键盘定位发送到ICT测试软件条码框中,然后进行ICT测试。如果没有,label状态栏变红,并提示“请进行测试前点检”,然后就先进行设置条码的ICT测试,判断扫描到txt_Barcode条码是否为获取到设置的良品或不良品条码,如输入的条码不符合,label状态栏变红,并提示“条码错误”,如输入的条码正确并为设置的良品条码,label状态栏变绿,并提示“请进行良品点检”,进行ICT测试,当发现某一文件夹中有良品测试报告生成,表示良品测试完成,测试完成后就提示“良品点检完成”。如扫描的是设置的不良品条码,则label状态栏变绿,并提示“请进行不良品点检”,进行ICT测试。当发现某一文件夹中有不良品测试报告生成,表示不良品测试完成。
时间: 2023-11-09 16:06:42 浏览: 49
这个功能可以分为几个模块来实现:
1. 定时器扫描数据库是否有设置的良品和不良品条码的当天ICT测试记录。
2. 如果有记录存在,更新label状态栏,并提示“已点检,请进行测试”,否则更新label状态栏,并提示“请进行测试前点检”。
3. 实现条码扫描后自动发送到ICT测试软件条码框中。
4. 实现ICT测试的功能,包括良品和不良品测试。
5. 实现良品点检和不良品点检的功能,包括判断条码是否为设置的良品或不良品条码。
6. 当发现某一文件夹中有良品或不良品测试报告生成,更新label状态栏,并提示“良品/不良品点检完成”。
下面是一个实现这个功能的C#窗体应用程序的示例代码:
```csharp
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.IO;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace BarcodeScannerApp
{
public partial class MainForm : Form
{
private string goodBarcodeFilePath = @"C:\good_barcodes.txt";
private string badBarcodeFilePath = @"C:\bad_barcodes.txt";
private string ictTestFolderPath = @"C:\ICT_Test_Results";
private Timer timer;
public MainForm()
{
InitializeComponent();
// 初始化定时器
timer = new Timer();
timer.Interval = 1000 * 60 * 10; // 每隔10分钟扫描一次数据库
timer.Tick += OnTimerTick;
timer.Start();
}
private void OnTimerTick(object sender, EventArgs e)
{
// 扫描数据库是否有设置的良品和不良品条码的当天ICT测试记录
bool hasGoodBarcodeRecord = CheckDatabaseForBarcode(goodBarcodeFilePath);
bool hasBadBarcodeRecord = CheckDatabaseForBarcode(badBarcodeFilePath);
if (hasGoodBarcodeRecord || hasBadBarcodeRecord)
{
// 更新label状态栏,并提示“已点检,请进行测试”
labelStatus.Text = "已点检,请进行测试";
labelStatus.ForeColor = Color.Green;
}
else
{
// 更新label状态栏,并提示“请进行测试前点检”
labelStatus.Text = "请进行测试前点检";
labelStatus.ForeColor = Color.Red;
}
}
// 扫描数据库是否有设置的良品或不良品条码的当天ICT测试记录
private bool CheckDatabaseForBarcode(string barcodeFilePath)
{
bool hasRecord = false;
// 读取条码文件
List<string> barcodes = new List<string>();
if (File.Exists(barcodeFilePath))
{
barcodes = File.ReadAllLines(barcodeFilePath).ToList();
}
// 扫描数据库
string connectionString = "your_db_connection_string_here";
using (SqlConnection connection = new SqlConnection(connectionString))
{
connection.Open();
string sql = "SELECT Barcode FROM ICT_test WHERE Date = @today AND Result = 'Pass'";
SqlCommand command = new SqlCommand(sql, connection);
command.Parameters.AddWithValue("@today", DateTime.Today);
using (SqlDataReader reader = command.ExecuteReader())
{
while (reader.Read())
{
string barcode = reader.GetString(0);
if (barcodes.Contains(barcode))
{
hasRecord = true;
break;
}
}
}
}
return hasRecord;
}
// 处理条码扫描事件
private void OnBarcodeScanned(string barcode)
{
// 发送条码到ICT测试软件条码框中
SendBarcodeToICTSoftware(barcode);
// 判断条码是否为设置的良品或不良品条码
bool isGoodBarcode = IsGoodBarcode(barcode);
bool isBadBarcode = IsBadBarcode(barcode);
if (!isGoodBarcode && !isBadBarcode)
{
// 更新label状态栏,并提示“条码错误”
labelStatus.Text = "条码错误";
labelStatus.ForeColor = Color.Red;
}
else if (isGoodBarcode)
{
// 更新label状态栏,并提示“请进行良品点检”
labelStatus.Text = "请进行良品点检";
labelStatus.ForeColor = Color.Green;
}
else if (isBadBarcode)
{
// 更新label状态栏,并提示“请进行不良品点检”
labelStatus.Text = "请进行不良品点检";
labelStatus.ForeColor = Color.Green;
}
}
// 发送条码到ICT测试软件条码框中
private void SendBarcodeToICTSoftware(string barcode)
{
// TODO: 实现发送条码到ICT测试软件条码框中的功能
}
// 判断条码是否为设置的良品条码
private bool IsGoodBarcode(string barcode)
{
// 读取良品条码文件
List<string> goodBarcodes = new List<string>();
if (File.Exists(goodBarcodeFilePath))
{
goodBarcodes = File.ReadAllLines(goodBarcodeFilePath).ToList();
}
return goodBarcodes.Contains(barcode);
}
// 判断条码是否为设置的不良品条码
private bool IsBadBarcode(string barcode)
{
// 读取不良品条码文件
List<string> badBarcodes = new List<string>();
if (File.Exists(badBarcodeFilePath))
{
badBarcodes = File.ReadAllLines(badBarcodeFilePath).ToList();
}
return badBarcodes.Contains(barcode);
}
// 处理ICT测试完成事件
private void OnICTTestCompleted(bool isGoodBarcode)
{
if (isGoodBarcode)
{
// 更新label状态栏,并提示“良品点检完成”
labelStatus.Text = "良品点检完成";
labelStatus.ForeColor = Color.Green;
// 检查某一文件夹中是否有良品测试报告生成
if (CheckFolderForICTTestResult(ictTestFolderPath, "Pass"))
{
// 更新label状态栏,并提示“良品点检完成”
labelStatus.Text = "良品点检完成";
labelStatus.ForeColor = Color.Green;
}
}
else
{
// 更新label状态栏,并提示“不良品点检完成”
labelStatus.Text = "不良品点检完成";
labelStatus.ForeColor = Color.Green;
// 检查某一文件夹中是否有不良品测试报告生成
if (CheckFolderForICTTestResult(ictTestFolderPath, "Fail"))
{
// 更新label状态栏,并提示“不良品点检完成”
labelStatus.Text = "不良品点检完成";
labelStatus.ForeColor = Color.Green;
}
}
}
// 检查某一文件夹中是否有ICT测试报告生成
private bool CheckFolderForICTTestResult(string folderPath, string testResult)
{
bool hasResult = false;
DirectoryInfo folder = new DirectoryInfo(folderPath);
foreach (FileInfo file in folder.GetFiles())
{
if (file.Extension == ".txt")
{
string[] lines = File.ReadAllLines(file.FullName);
if (lines.Length >= 2 && lines[1].StartsWith("Result:"))
{
string result = lines[1].Substring(7).Trim();
if (result == testResult)
{
hasResult = true;
break;
}
}
}
}
return hasResult;
}
// 处理扫描按钮点击事件
private void btnScan_Click(object sender, EventArgs e)
{
string barcode = txtBarcode.Text.Trim();
OnBarcodeScanned(barcode);
}
// 处理ICT测试完成事件
private void OnICTTestCompleted(bool isGoodBarcode)
{
if (isGoodBarcode)
{
// 更新label状态栏,并提示“良品点检完成”
labelStatus.Text = "良品点检完成";
labelStatus.ForeColor = Color.Green;
// 检查某一文件夹中是否有良品测试报告生成
if (CheckFolderForICTTestResult(ictTestFolderPath, "Pass"))
{
// 更新label状态栏,并提示“良品点检完成”
labelStatus.Text = "良品点检完成";
labelStatus.ForeColor = Color.Green;
}
}
else
{
// 更新label状态栏,并提示“不良品点检完成”
labelStatus.Text = "不良品点检完成";
labelStatus.ForeColor = Color.Green;
// 检查某一文件夹中是否有不良品测试报告生成
if (CheckFolderForICTTestResult(ictTestFolderPath, "Fail"))
{
// 更新label状态栏,并提示“不良品点检完成”
labelStatus.Text = "不良品点检完成";
labelStatus.ForeColor = Color.Green;
}
}
}
}
}
```
相关推荐
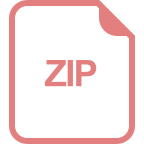





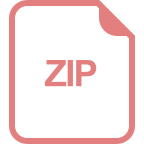
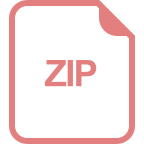
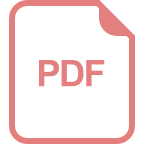
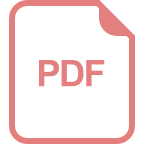
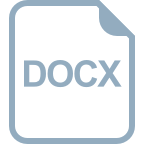