我需要一段httpClient实现视频分片上传功能的代码
时间: 2024-06-10 09:06:31 浏览: 101
好的,以下是一个基于HttpClient的视频分片上传示例代码:
```
import java.io.File;
import java.io.IOException;
import java.io.InputStream;
import org.apache.http.HttpEntity;
import org.apache.http.HttpResponse;
import org.apache.http.HttpStatus;
import org.apache.http.client.ClientProtocolException;
import org.apache.http.client.HttpClient;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.entity.mime.HttpMultipartMode;
import org.apache.http.entity.mime.MultipartEntityBuilder;
import org.apache.http.entity.mime.content.FileBody;
import org.apache.http.entity.mime.content.InputStreamBody;
import org.apache.http.entity.mime.content.StringBody;
import org.apache.http.impl.client.HttpClientBuilder;
public class VideoUploader {
private static final int BUFFER_SIZE = 4096;
public static void main(String[] args) throws IOException {
String filePath = "/path/to/video.mp4";
String uploadUrl = "https://example.com/upload";
int chunkSize = 1024 * 1024; // 1MB chunks
// Get file information
File videoFile = new File(filePath);
String fileName = videoFile.getName();
long fileSize = videoFile.length();
// Create HTTP client
HttpClient httpClient = HttpClientBuilder.create().build();
// Upload file in chunks
int chunkIndex = 0;
long bytesUploaded = 0;
while (bytesUploaded < fileSize) {
long bytesRemaining = fileSize - bytesUploaded;
int chunkLength = (int) Math.min(chunkSize, bytesRemaining);
byte[] chunkBuffer = new byte[chunkLength];
// Read chunk from file
InputStream inputStream = new InputStream() {
private int bytesRead = 0;
@Override
public int read() throws IOException {
if (bytesRead < chunkLength) {
int b = System.in.read();
bytesRead++;
if (b == -1) {
return -1;
} else {
chunkBuffer[bytesRead - 1] = (byte) b;
return b;
}
} else {
return -1;
}
}
};
// Create multipart entity
MultipartEntityBuilder builder = MultipartEntityBuilder.create();
builder.setMode(HttpMultipartMode.BROWSER_COMPATIBLE);
builder.addPart("file", new InputStreamBody(inputStream, fileName + ".part" + chunkIndex));
builder.addPart("chunkIndex", new StringBody(Integer.toString(chunkIndex)));
builder.addPart("chunkSize", new StringBody(Integer.toString(chunkSize)));
builder.addPart("totalSize", new StringBody(Long.toString(fileSize)));
HttpEntity entity = builder.build();
// Create HTTP post request
HttpPost httpPost = new HttpPost(uploadUrl);
httpPost.setEntity(entity);
// Execute HTTP request
HttpResponse response = httpClient.execute(httpPost);
if (HttpStatus.SC_OK == response.getStatusLine().getStatusCode()) {
System.out.println("Uploaded " + chunkLength + " bytes");
bytesUploaded += chunkLength;
chunkIndex++;
} else {
System.err.println("Error uploading file: " + response.getStatusLine().getStatusCode());
break;
}
}
System.out.println("Finished uploading file");
}
}
```
注意,这段代码仅为示例代码,不能直接运行。您需要根据实际情况修改代码,并确保在上传视频时遵守相关法规和规定。
阅读全文
相关推荐
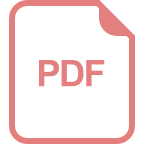
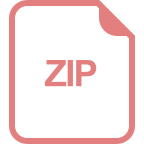
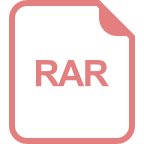
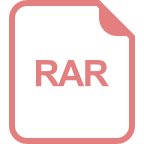
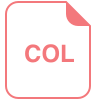
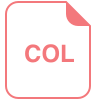
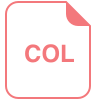


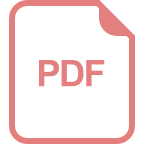
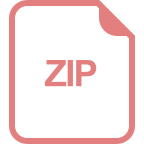
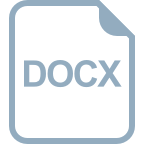
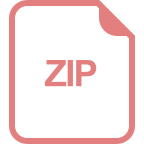