小型教务管理系统设计c语言代码
时间: 2023-08-29 22:11:24 浏览: 157
以下是一个简单的小型教务管理系统的 C 语言代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 学生信息结构体
typedef struct Student {
char name[20];
char id[10];
float score;
} Student;
// 学生信息链表节点结构体
typedef struct StudentNode {
Student data;
struct StudentNode *next;
} StudentNode;
// 创建新的学生信息节点
StudentNode *createStudentNode(Student student) {
StudentNode *node = (StudentNode *)malloc(sizeof(StudentNode));
node->data = student;
node->next = NULL;
return node;
}
// 添加学生信息到链表中
void addStudent(StudentNode **head, Student student) {
StudentNode *node = createStudentNode(student);
if (*head == NULL) {
*head = node;
} else {
StudentNode *current = *head;
while (current->next != NULL) {
current = current->next;
}
current->next = node;
}
}
// 显示学生信息
void showStudent(Student student) {
printf("Name: %s\n", student.name);
printf("ID: %s\n", student.id);
printf("Score: %.2f\n", student.score);
}
// 显示所有学生信息
void showAllStudents(StudentNode *head) {
if (head == NULL) {
printf("No students found.\n");
} else {
StudentNode *current = head;
while (current != NULL) {
showStudent(current->data);
current = current->next;
}
}
}
// 查找学生信息
StudentNode *findStudent(StudentNode *head, char *id) {
StudentNode *current = head;
while (current != NULL) {
if (strcmp(current->data.id, id) == 0) {
return current;
}
current = current->next;
}
return NULL;
}
// 删除学生信息
void deleteStudent(StudentNode **head, char *id) {
if (*head == NULL) {
printf("No students found.\n");
} else {
StudentNode *current = *head;
StudentNode *previous = NULL;
while (current != NULL) {
if (strcmp(current->data.id, id) == 0) {
if (previous == NULL) {
*head = current->next;
} else {
previous->next = current->next;
}
free(current);
printf("Student deleted.\n");
return;
}
previous = current;
current = current->next;
}
printf("No students found with ID: %s\n", id);
}
}
int main() {
StudentNode *head = NULL;
// 添加学生信息
addStudent(&head, (Student){"Alice", "1001", 90.5});
addStudent(&head, (Student){"Bob", "1002", 85.0});
addStudent(&head, (Student){"Charlie", "1003", 92.5});
// 显示所有学生信息
showAllStudents(head);
// 查找学生信息
StudentNode *student = findStudent(head, "1002");
if (student != NULL) {
printf("Found student:\n");
showStudent(student->data);
} else {
printf("Student not found.\n");
}
// 删除学生信息
deleteStudent(&head, "1003");
// 显示所有学生信息
showAllStudents(head);
return 0;
}
```
这个教务管理系统使用了链表来存储学生信息,并提供了添加、显示、查找和删除学生信息的功能。当然,这只是一个简单的示例,实际的教务管理系统需要更复杂的功能和更多的数据处理。
阅读全文
相关推荐
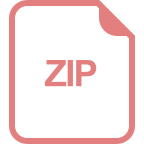
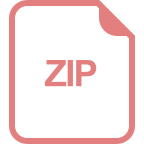
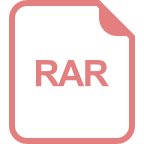

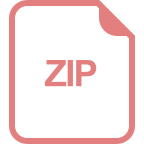
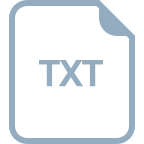
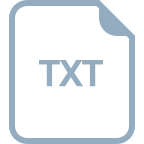
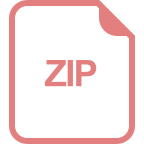
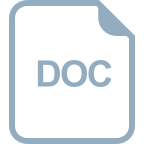
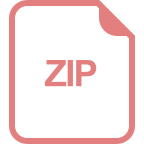
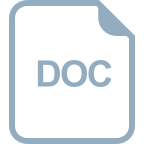
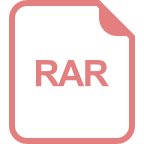
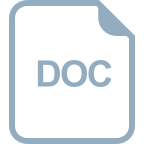
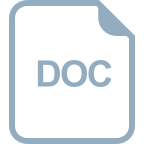
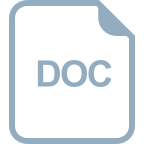
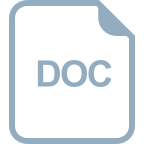
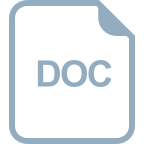
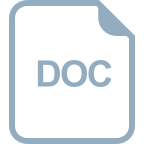