给出基于a*算法的路径规划代码,要求使用图像表示结果,并含有注释
时间: 2024-02-21 14:57:14 浏览: 86
好的,让我来给你展示一个基于a*算法的路径规划代码的示例。我将使用Python语言,使用matplotlib库来绘制图像。代码如下:
```python
import numpy as np
import matplotlib.pyplot as plt
from queue import PriorityQueue
# 定义地图大小
ROWS = 50
COLS = 50
# 定义起点和终点
start = (0, 0)
end = (ROWS-1, COLS-1)
# 定义障碍物
obstacles = [(1, 1), (2, 2), (3, 3), (4, 4), (5, 5), (6, 6), (7, 7), (8, 8), (9, 9)]
# 定义启发函数,这里使用曼哈顿距离
def heuristic(a, b):
return np.abs(a[0] - b[0]) + np.abs(a[1] - b[1])
# 定义可行函数,判断某个点是否是合法的
def is_valid(x, y):
if x < 0 or y < 0 or x >= ROWS or y >= COLS:
return False
if (x, y) in obstacles:
return False
return True
# 定义a*算法
def astar(start, end):
# 初始化起点和终点
start_node = (start, 0)
end_node = (end, float('inf'))
frontier = PriorityQueue()
frontier.put(start_node, 0)
came_from = {}
cost_so_far = {}
came_from[start] = None
cost_so_far[start] = 0
# 开始搜索
while not frontier.empty():
current_node = frontier.get()
current = current_node[0]
# 到达终点
if current == end:
break
# 遍历相邻节点
for dx, dy in [(0, 1), (1, 0), (0, -1), (-1, 0)]:
next_node = (current[0]+dx, current[1]+dy)
# 判断是否为合法节点
if not is_valid(next_node[0], next_node[1]):
continue
# 计算到起点的距离
new_cost = cost_so_far[current] + 1
# 如果未被访问过,或者到起点的距离更短,则更新信息
if next_node not in cost_so_far or new_cost < cost_so_far[next_node]:
cost_so_far[next_node] = new_cost
priority = new_cost + heuristic(end, next_node)
frontier.put((next_node, priority))
came_from[next_node] = current
# 反向回溯路径
path = []
node = end
while node != start:
path.append(node)
node = came_from[node]
path.append(start)
path.reverse()
return path
# 绘制地图
def draw_map(path):
# 初始化地图
world = np.zeros((ROWS, COLS))
# 绘制障碍物
for obstacle in obstacles:
world[obstacle[0], obstacle[1]] = 1
# 绘制路径
for node in path:
world[node[0], node[1]] = 2
# 绘制起点和终点
world[start[0], start[1]] = 3
world[end[0], end[1]] = 4
# 绘制地图
plt.imshow(world)
plt.axis('off')
plt.show()
# 运行a*算法
path = astar(start, end)
# 绘制地图
draw_map(path)
```
这个示例代码将生成一个50x50的地图,其中起点为(0,0),终点为(49,49),障碍物为(1,1)到(9,9)的九个点。算法使用曼哈顿距离作为启发函数,使用优先队列来维护搜索队列。最后,使用matplotlib库将结果绘制成图像,其中障碍物为黑色,路径为蓝色,起点为绿色,终点为红色。
希望这个示例代码能够帮助你理解基于a*算法的路径规划,并帮助你更好地理解如何使用图像表示结果。
阅读全文
相关推荐
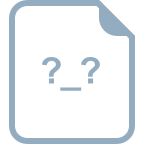
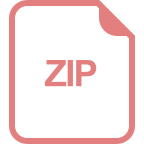
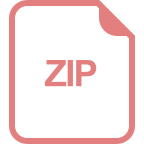
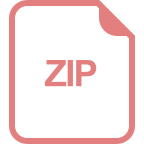
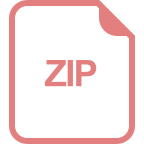
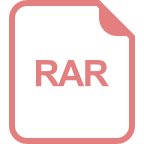
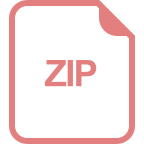
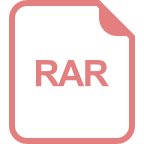
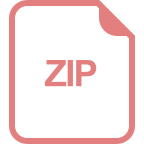
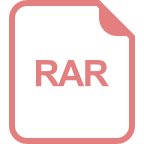
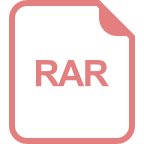
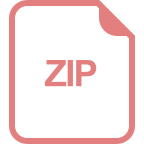
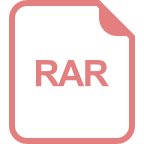
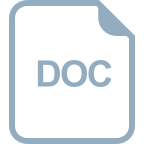
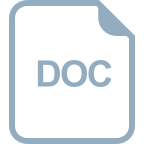
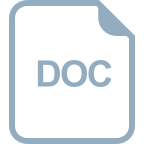
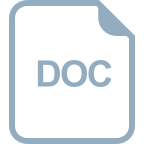