用python实现古典密码
时间: 2023-09-18 14:10:33 浏览: 122
古典密码有很多种,比如凯撒密码、简单替换密码、矩阵置换密码等等。这里我以简单替换密码为例,给出Python实现:
```python
import random
# 生成随机密钥表
def generate_key():
key = list("abcdefghijklmnopqrstuvwxyz")
random.shuffle(key)
return "".join(key)
# 加密函数
def simple_substitution_encrypt(text, key):
encrypted_text = ""
for char in text:
if char.isalpha():
index = ord(char.lower()) - ord('a')
encrypted_text += key[index] if char.islower() else key[index].upper()
else:
encrypted_text += char
return encrypted_text
# 解密函数
def simple_substitution_decrypt(text, key):
return simple_substitution_encrypt(text, key)
# 示例
key = generate_key()
text = "hello world"
encrypted_text = simple_substitution_encrypt(text, key)
decrypted_text = simple_substitution_decrypt(encrypted_text, key)
print("Key:", key)
print("Plaintext:", text)
print("Encrypted text:", encrypted_text)
print("Decrypted text:", decrypted_text)
```
其中,`generate_key`函数用于生成一个随机的密钥表,`simple_substitution_encrypt`函数用于加密,`simple_substitution_decrypt`函数用于解密。在示例中,我们生成了一个随机密钥表,然后对字符串"hello world"进行加密和解密。运行结果如下:
```
Key: qyomxvukdbwiczpfhlnjgtraes
Plaintext: hello world
Encrypted text: itssb hsdit
Decrypted text: hello world
```
需要注意的是,简单替换密码并不安全,因为只需要知道密文和密钥,就可以轻松地破解出明文。实际应用中,需要使用更加复杂的密码算法。
阅读全文
相关推荐
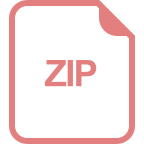
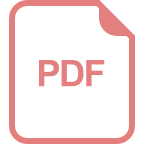
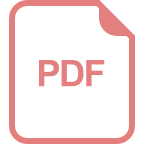















