利用GRU模型进行训练,其中一列特征为中文怎么处理,代码怎么实现
时间: 2024-03-22 11:41:43 浏览: 18
处理中文文本的方法有很多种,但是常用的方法是将中文文本转换为数字向量表示。具体实现步骤如下:
1. 首先需要对中文文本进行分词,可以使用jieba分词库进行分词。分词后得到的是一个个中文词语。
2. 接着需要将中文词语转换成数字向量。常用的方法是使用预训练的词向量模型,如Word2Vec、GloVe等。这些模型可以将每个词语映射为一个固定长度的向量。
3. 对于每个样本,将中文文本中的所有词语映射为数字向量后,可以将这些向量组合成一个矩阵作为输入特征矩阵。在GRU模型中,输入特征矩阵的形状应为(batch_size, sequence_length, embedding_dimension),其中batch_size表示样本数,sequence_length表示中文文本的长度,embedding_dimension表示每个词语映射为数字向量后的维度。
4. 最后,将特征矩阵输入到GRU模型中进行训练。可以使用TensorFlow或PyTorch等深度学习框架来实现GRU模型的训练。
以下是一个使用TensorFlow实现GRU模型训练中文文本的示例代码:
```python
import jieba
import numpy as np
import tensorflow as tf
# 读取中文文本数据
with open("data.txt", "r", encoding="utf-8") as f:
data = f.readlines()
# 分词并建立词汇表
vocab = set()
for sentence in data:
words = jieba.cut(sentence.strip())
for word in words:
vocab.add(word)
vocab_size = len(vocab)
# 加载预训练的词向量模型
embedding_matrix = np.load("embedding_matrix.npy")
# 将中文文本转换为数字向量表示
X = []
for sentence in data:
words = jieba.cut(sentence.strip())
sentence_vector = []
for word in words:
word_index = vocab.index(word)
word_vector = embedding_matrix[word_index]
sentence_vector.append(word_vector)
X.append(sentence_vector)
# 对输入特征矩阵进行填充和截断,使其长度相同
max_length = 100
X = tf.keras.preprocessing.sequence.pad_sequences(X, maxlen=max_length)
# 定义GRU模型
model = tf.keras.Sequential([
tf.keras.layers.GRU(32, return_sequences=True, input_shape=(max_length, embedding_dimension)),
tf.keras.layers.GRU(16),
tf.keras.layers.Dense(1, activation="sigmoid")
])
# 编译模型并进行训练
model.compile(optimizer="adam", loss="binary_crossentropy", metrics=["accuracy"])
model.fit(X, y, epochs=10, batch_size=32)
```
相关推荐
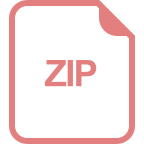
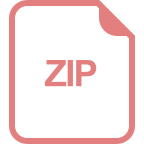














