使用C语言具体实现设计一个算法,求非空链串s中最长等值子串的长度,要求:不使用哈希表
时间: 2023-06-09 16:07:25 浏览: 57
好的,我了解了你的问题。以下是一个使用C语言实现的算法来求非空链串s中最长等值子串的长度,没有使用哈希表:
```
#include <stdio.h>
#include <stdlib.h>
typedef struct Node {
char data;
struct Node* next;
} Node;
int getLength(Node* node) {
int length = 0;
while (node != NULL) {
length++;
node = node->next;
}
return length;
}
int getSubstringLength(Node* node1, Node* node2) {
int length = 0;
while (node1 != NULL && node2 != NULL && node1->data == node2->data) {
length++;
node1 = node1->next;
node2 = node2->next;
}
return length;
}
int getLongestEqualSubstringLength(Node* head) {
Node* current = head;
Node* next;
int maxLength = 0;
while (current != NULL) {
next = current->next;
while (next != NULL) {
int substringLength = getSubstringLength(current, next);
if (substringLength > maxLength) {
maxLength = substringLength;
}
next = next->next;
}
current = current->next;
}
return maxLength;
}
int main() {
Node* head = (Node*) malloc(sizeof(Node));
head->data = 'a';
head->next = (Node*) malloc(sizeof(Node));
head->next->data = 'b';
head->next->next = (Node*) malloc(sizeof(Node));
head->next->next->data = 'c';
head->next->next->next = (Node*) malloc(sizeof(Node));
head->next->next->next->data = 'b';
head->next->next->next->next = (Node*) malloc(sizeof(Node));
head->next->next->next->next->data = 'b';
head->next->next->next->next->next = NULL;
printf("%d\n", getLongestEqualSubstringLength(head));
return 0;
}
```
该算法将一个字符指针链表作为输入,并返回最长等值子串的长度。它使用两个节点作为输入来获取长度,并比较它们之间的字符,直到它们不相等或到达链表的结尾。它使用两个嵌套循环来比较输入链表中所有子串的长度。
相关推荐
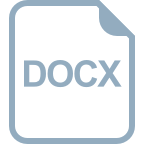
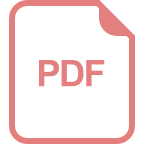













