qcustomplot 通过鼠标轨迹画矩形框
时间: 2023-10-29 12:05:44 浏览: 164
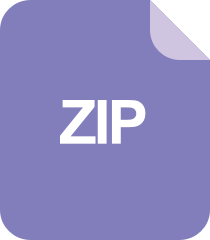
qt界面编程,画矩形框

要通过鼠标轨迹画矩形框,可以结合使用QCustomPlot的鼠标事件和QCPItemRect类。可以按照以下步骤进行操作:
1. 创建一个QCPItemRect对象:
```
QCPItemRect *rect = new QCPItemRect(customPlot);
rect->setPen(QPen(Qt::red));
rect->setBrush(QBrush(Qt::blue));
```
2. 实现鼠标事件:
```
void MainWindow::mousePressEvent(QMouseEvent *event)
{
if (event->button() == Qt::LeftButton)
{
rect->topLeft->setCoords(ui->customPlot->xAxis->pixelToCoord(event->pos().x()), ui->customPlot->yAxis->pixelToCoord(event->pos().y()));
rect->bottomRight->setCoords(ui->customPlot->xAxis->pixelToCoord(event->pos().x()), ui->customPlot->yAxis->pixelToCoord(event->pos().y()));
rect->setVisible(true);
ui->customPlot->replot();
}
}
void MainWindow::mouseMoveEvent(QMouseEvent *event)
{
if (event->buttons() == Qt::LeftButton)
{
rect->bottomRight->setCoords(ui->customPlot->xAxis->pixelToCoord(event->pos().x()), ui->customPlot->yAxis->pixelToCoord(event->pos().y()));
ui->customPlot->replot();
}
}
void MainWindow::mouseReleaseEvent(QMouseEvent *event)
{
if (event->button() == Qt::LeftButton)
{
rect->setVisible(false);
ui->customPlot->replot();
}
}
```
其中,鼠标按下时记录矩形框左上角的坐标,鼠标移动时更新矩形框右下角的坐标并重新绘制,鼠标释放时隐藏矩形框并重新绘制。
完整的代码示例:
```
void MainWindow::mousePressEvent(QMouseEvent *event)
{
if (event->button() == Qt::LeftButton)
{
rect->topLeft->setCoords(ui->customPlot->xAxis->pixelToCoord(event->pos().x()), ui->customPlot->yAxis->pixelToCoord(event->pos().y()));
rect->bottomRight->setCoords(ui->customPlot->xAxis->pixelToCoord(event->pos().x()), ui->customPlot->yAxis->pixelToCoord(event->pos().y()));
rect->setVisible(true);
ui->customPlot->replot();
}
}
void MainWindow::mouseMoveEvent(QMouseEvent *event)
{
if (event->buttons() == Qt::LeftButton)
{
rect->bottomRight->setCoords(ui->customPlot->xAxis->pixelToCoord(event->pos().x()), ui->customPlot->yAxis->pixelToCoord(event->pos().y()));
ui->customPlot->replot();
}
}
void MainWindow::mouseReleaseEvent(QMouseEvent *event)
{
if (event->button() == Qt::LeftButton)
{
rect->setVisible(false);
ui->customPlot->replot();
}
}
void MainWindow::setupPlot()
{
// ...
rect = new QCPItemRect(ui->customPlot);
rect->setPen(QPen(Qt::red));
rect->setBrush(QBrush(Qt::blue));
rect->setVisible(false);
}
```
阅读全文
相关推荐
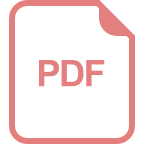
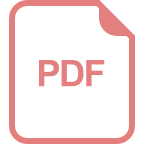
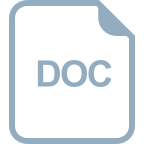

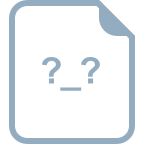
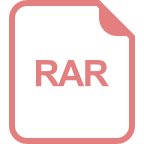
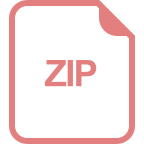
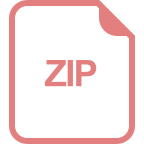
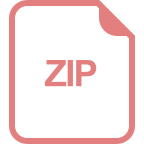
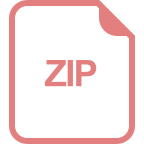
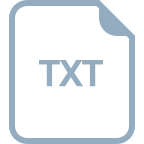
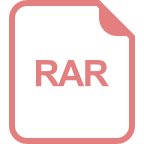