查询某日借书情况 c语言编程,急求程序!!!简单图书馆借/还书管理子系统...
时间: 2023-06-18 13:06:59 浏览: 63
以下是一个简单的C语言程序,可以查询某日借书情况:
```c
#include <stdio.h>
#include <string.h>
struct Book {
char title[50];
char author[50];
int id;
int borrowed;
char borrowed_date[20];
};
void display(struct Book b[], int n) {
int i;
printf("\nTitle\t\tAuthor\t\tID\tBorrowed\tBorrowed Date\n");
for(i = 0; i < n; i++) {
printf("%s\t\t%s\t\t%d\t%d\t\t%s\n", b[i].title, b[i].author, b[i].id, b[i].borrowed, b[i].borrowed_date);
}
}
void search(struct Book b[], int n, char date[]) {
int i, count = 0;
printf("\nBooks borrowed on %s:\n", date);
for(i = 0; i < n; i++) {
if(strcmp(b[i].borrowed_date, date) == 0) {
printf("%s\n", b[i].title);
count++;
}
}
if(count == 0) {
printf("No books borrowed on %s\n", date);
}
}
int main() {
int n, i;
char date[20];
printf("Enter the number of books: ");
scanf("%d", &n);
struct Book b[n];
for(i = 0; i < n; i++) {
printf("Enter the details of book %d:\n", i+1);
printf("Title: ");
scanf("%s", b[i].title);
printf("Author: ");
scanf("%s", b[i].author);
printf("ID: ");
scanf("%d", &b[i].id);
printf("Borrowed (1 for yes, 0 for no): ");
scanf("%d", &b[i].borrowed);
if(b[i].borrowed == 1) {
printf("Borrowed Date: ");
scanf("%s", b[i].borrowed_date);
}
}
display(b, n);
printf("\nEnter the date to search (DD/MM/YYYY): ");
scanf("%s", date);
search(b, n, date);
return 0;
}
```
该程序使用结构体存储图书的信息,并提供了两个函数:`display()` 用于显示所有图书的信息,`search()` 用于查询某日借书情况。在主函数中,用户需要输入图书的数量以及每本图书的详细信息。用户还需要输入查询的日期。程序将在所有借阅的图书中查找该日期,并输出相应的结果。
注意:该程序只是一个简单的示例,实际使用时需要根据实际情况进行修改和扩展。
相关推荐
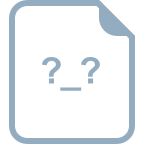














